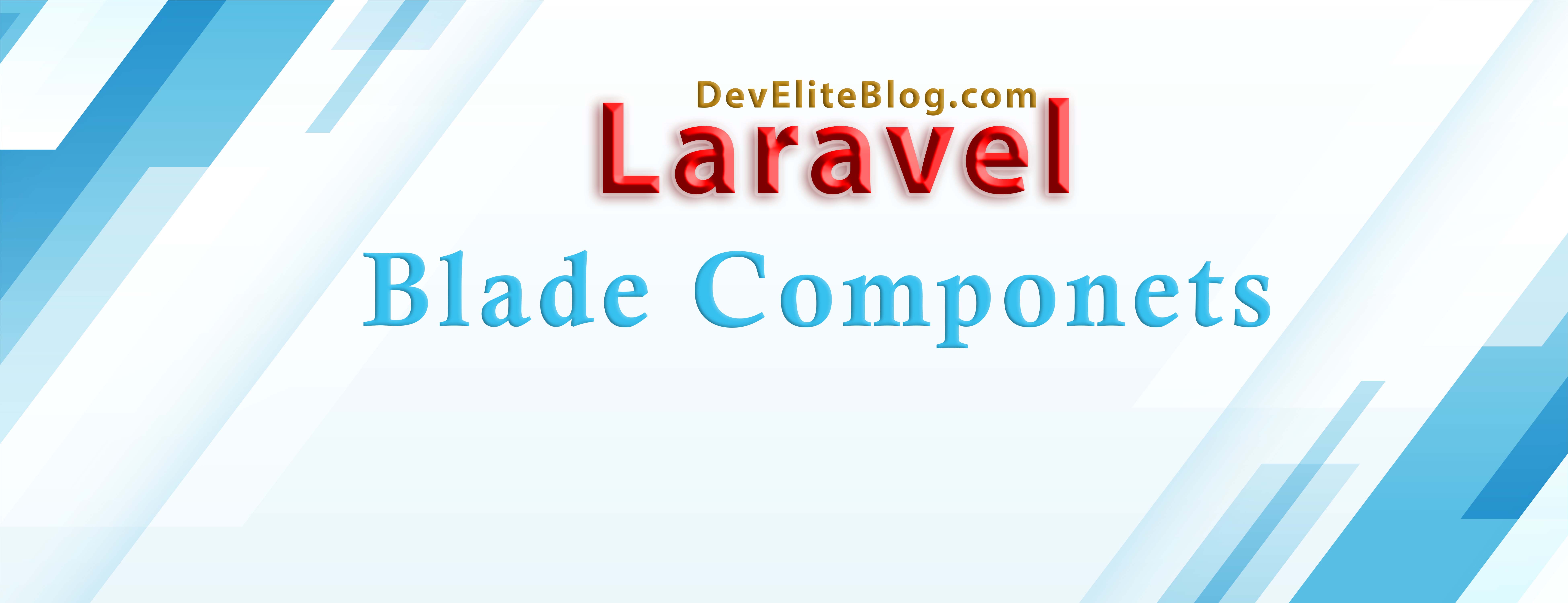
# Blade Components
Laravel Blade Components cung cấp một cách tiện lợi để xây dựng các phần tử HTML tái sử dụng, giúp bạn tổ chức mã nguồn một cách rõ ràng và hiệu quả hơn. Blade Components cho phép bạn tách biệt logic và giao diện, giúp việc phát triển và bảo trì ứng dụng trở nên dễ dàng hơn.
1. Tạo Blade Components
Tạo Component Bằng Lệnh Artisan
Bạn có thể sử dụng lệnh Artisan để tạo một component:
php artisan make:component Alert
Lệnh này sẽ tạo hai file:
- Một class component tại
app/View/Components/Alert.php
- Một view Blade tại
resources/views/components/alert.blade.php
File Class Component
File: app/View/Components/Alert.php
<?php
namespace App\View\Components;
use Illuminate\View\Component;
class Alert extends Component
{
public $type;
/**
* Create a new component instance.
*
* @return void
*/
public function __construct($type)
{
$this->type = $type;
}
/**
* Get the view / contents that represent the component.
*
* @return \Illuminate\Contracts\View\View|\Closure|string
*/
public function render()
{
return view('components.alert');
}
}
File View Component
File: resources/views/components/alert.blade.php
<div class="alert alert-{{ $type }}">
{{ $slot }}
</div>
2. Sử Dụng Blade Components
Nhúng Component Trong View
Bạn có thể nhúng component trong view Blade bằng cú pháp <x-component-name>
:
File: resources/views/welcome.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Welcome</title>
</head>
<body>
<x-alert type="success">
This is a success alert.
</x-alert>
<x-alert type="danger">
This is a danger alert.
</x-alert>
</body>
</html>
3. Truyền Dữ Liệu Cho Component
Bạn có thể truyền dữ liệu cho component thông qua các thuộc tính:
File: app/View/Components/Alert.php
public $type;
public function __construct($type)
{
$this->type = $type;
}
File: resources/views/components/alert.blade.php
<div class="alert alert-{{ $type }}">
{{ $slot }}
</div>
File: resources/views/welcome.blade.php
<x-alert type="success">
This is a success alert.
</x-alert>
<x-alert type="danger">
This is a danger alert.
</x-alert>
4. Slots
Slots cho phép bạn xác định các phần nội dung động trong component. Mặc định, tất cả nội dung được truyền vào component sẽ nằm trong biến $slot
.
Slots Mặc Định
File: resources/views/components/alert.blade.php
<div class="alert alert-{{ $type }}">
{{ $slot }}
</div>
File: resources/views/welcome.blade.php
<x-alert type="success">
This is a success alert.
</x-alert>
Named Slots
Bạn có thể tạo nhiều slots với tên khác nhau.
File: resources/views/components/alert.blade.php
<div class="alert alert-{{ $type }}">
<div class="alert-title">{{ $title }}</div>
{{ $slot }}
</div>
File: resources/views/welcome.blade.php
<x-alert type="success">
<x-slot name="title">
Success
</x-slot>
This is a success alert.
</x-alert>
5. Inline Components
Nếu component của bạn đơn giản và không yêu cầu logic phức tạp, bạn có thể sử dụng Inline Components mà không cần tạo file class riêng.
File: resources/views/components/button.blade.php
<button {{ $attributes->merge(['class' => 'btn btn-primary']) }}>
{{ $slot }}
</button>
File: resources/views/welcome.blade.php
<x-button>
Click Me
</x-button>
Tóm Tắt
- Tạo Component: Sử dụng lệnh Artisan để tạo component.
- Nhúng Component: Sử dụng cú pháp
<x-component-name>
để nhúng component trong view. - Truyền Dữ Liệu: Truyền dữ liệu cho component thông qua các thuộc tính.
- Slots: Sử dụng slots để xác định nội dung động trong component.
- Inline Components: Sử dụng Inline Components cho các component đơn giản.
Blade Components giúp mã nguồn của bạn trở nên sạch sẽ, dễ đọc và dễ bảo trì hơn. Việc tách biệt giao diện và logic giúp bạn tập trung vào từng khía cạnh riêng biệt của ứng dụng, làm cho quá trình phát triển trở nên hiệu quả hơn.
Danh mục
Bài viết liên quan
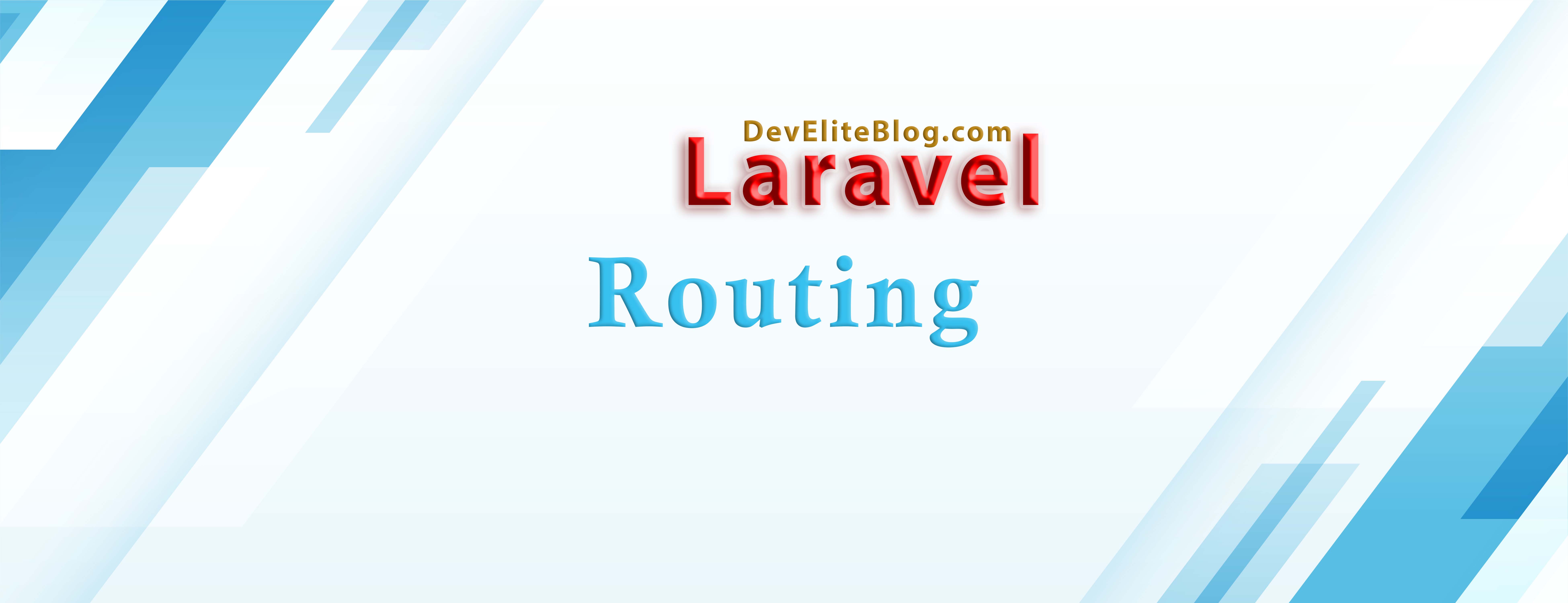
Routing
Author: | ADMIN |
---|
# Basic Routing
1. Khái niệm về Route
Route trong Laravel là cách bạn định nghĩa URL cho ứng dụng của mình. Route giúp bạn chỉ định URL nào sẽ gọi đến Controller nào hoặc thực hiện hành động gì.
2. Định nghĩa Route Cơ Bản
Tất cả các route của Laravel được định nghĩa trong các file nằm trong thư mục routes
. Các file này được tự động tải bởi App\Providers\RouteServiceProvider
của ứng dụng của bạn. Có bốn file route chính:
web.php
: Định nghĩa các route cho web application.api.php
: Định nghĩa các route cho API.console.php
: Định nghĩa các route cho console commands.channels.php
: Định nghĩa các route cho event broadcasting channels.
Định nghĩa route trong web.php
Dưới đây là một ví dụ đơn giản về cách định nghĩa một route trong file web.php
:
Route::get('/', function () {
return view('welcome');
});
Trong ví dụ trên:
Route::get('/')
: Định nghĩa một route sử dụng phương thức GET cho URL/
.function () { return view('welcome'); }
: Định nghĩa hành động sẽ thực hiện khi người dùng truy cập vào URL/
. Ở đây, Laravel sẽ trả về viewwelcome
.
3. Các Phương Thức Route
Laravel hỗ trợ nhiều phương thức HTTP khác nhau, bao gồm:
GET
: Dùng để truy xuất dữ liệu từ server.POST
: Dùng để gửi dữ liệu lên server.PUT
: Dùng để cập nhật dữ liệu trên server.PATCH
: Dùng để cập nhật một phần tài nguyên trên server. Tương tự nhưPUT
nhưng chỉ cập nhật các phần cụ thể của tài nguyên.DELETE
: Dùng để xóa dữ liệu từ server.OPTIONS
: Dùng để truy vấn các phương thức HTTP mà server hỗ trợ cho một URL cụ thể. Thường được sử dụng trong các ứng dụng RESTful API để kiểm tra các tùy chọn giao tiếp.
Ví dụ về các phương thức khác nhau:
Route::get('/products', function () {
return 'Get all products';
});
Route::post('/products', function () {
return 'Create a new product';
});
Route::put('/products/{id}', function ($id) {
return 'Update the product with ID ' . $id;
});
Route::patch('/products/{id}', function ($id) {
return 'Partially update the product with ID ' . $id;
});
Route::delete('/products/{id}', function ($id) {
return 'Delete the product with ID ' . $id;
});
Route::options('/products', function () {
return response()->json(['GET', 'POST', 'PUT', 'PATCH', 'DELETE']);
});
4. Route với Tham Số
Bạn có thể định nghĩa route có tham số như sau:
Route::get('/user/{id}', function ($id) {
return 'User '.$id;
});
Trong ví dụ này, {id}
là một tham số động. Khi người dùng truy cập vào URL user/1
, Laravel sẽ gán giá trị 1
vào biến $id
và trả về User 1
.
5. Route Groups
Bạn có thể nhóm các route lại với nhau bằng cách sử dụng Route::group
. Điều này giúp bạn dễ dàng áp dụng middleware hoặc tiền tố URL cho một nhóm route.
Route::group(['prefix' => 'admin'], function () {
Route::get('/users', function () {
// Matches The "/admin/users" URL
});
Route::get('/settings', function () {
// Matches The "/admin/settings" URL
});
});
Trong ví dụ trên, tất cả các route trong nhóm này sẽ có tiền tố admin
.
6. Middleware
Middleware là các lớp trung gian mà HTTP request phải đi qua trước khi đến Controller. Bạn có thể áp dụng middleware cho route như sau:
Route::get('/profile', function () {
// Only authenticated users may enter...
})->middleware('auth');
Trong ví dụ này, middleware auth
sẽ kiểm tra xem người dùng có được xác thực hay không trước khi cho phép truy cập vào route /profile
.
Laravel cung cấp hai nhóm middleware chính cho các file route:
- web middleware group: Được áp dụng cho các route trong
routes/web.php
. Middleware nhóm này bao gồm các tính năng như:- Session state
- CSRF protection
- Cookie encryption
- ...
- api middleware group: Được áp dụng cho các route trong
routes/api.php
. Middleware nhóm này bao gồm các tính năng như:- Stateless (không trạng thái)
- Token-based authentication
- Rate limiting
- ...
7. CSRF Protection
Hãy nhớ rằng, bất kỳ biểu mẫu HTML nào trỏ tới các route sử dụng phương thức POST, PUT, PATCH, hoặc DELETE được định nghĩa trong file route web cần phải bao gồm trường token CSRF. Nếu không, yêu cầu sẽ bị từ chối. Bạn có thể đọc thêm về bảo vệ CSRF trong tài liệu CSRF:
<form method="POST" action="/profile">
@csrf
<!-- Các trường input khác -->
<button type="submit">Submit</button>
</form>
Việc bảo vệ CSRF rất quan trọng để ngăn chặn các tấn công giả mạo yêu cầu từ trang chéo, đảm bảo rằng các yêu cầu tới server đến từ nguồn đáng tin cậy.
8. Redirect Routes
Nếu bạn định nghĩa một route để chuyển hướng đến một URI khác, bạn có thể sử dụng phương thức Route::redirect
. Phương thức này cung cấp một cách tắt thuận tiện để bạn không phải định nghĩa một route hoặc controller đầy đủ cho việc thực hiện chuyển hướng đơn giản.
Ví dụ:
Route::redirect('/here', '/there');
Mặc định, Route::redirect
sẽ trả về mã trạng thái 302. Mã trạng thái 302 cho biết rằng tài nguyên đã được di chuyển tạm thời đến một vị trí mới.
Tuỳ Chỉnh Mã Trạng Thái
Bạn có thể tuỳ chỉnh mã trạng thái bằng cách sử dụng tham số thứ ba tùy chọn:
Route::redirect('/here', '/there', 301);
Trong ví dụ này, mã trạng thái 301 được sử dụng để chỉ ra rằng tài nguyên đã được di chuyển vĩnh viễn đến một vị trí mới.
Sử Dụng Route::permanentRedirect
Hoặc, bạn có thể sử dụng phương thức Route::permanentRedirect
để trả về mã trạng thái 301:
Route::permanentRedirect('/here', '/there');
9. The Route List
Lệnh Artisan route:list
là một công cụ mạnh mẽ giúp bạn dễ dàng xem tất cả các route đã được định nghĩa trong ứng dụng của bạn. Dưới đây là các cách sử dụng lệnh route:list
cùng với giải thích chi tiết:
Hiển Thị Danh Sách Các Route
Để xem tất cả các route được định nghĩa trong ứng dụng của bạn, bạn có thể sử dụng lệnh:
php artisan route:list
Lệnh này hiển thị một bảng tổng quan của tất cả các route trong ứng dụng, bao gồm các thông tin như phương thức HTTP, URI, tên route, và hành động (action) của route đó. Đây là cách nhanh chóng để bạn kiểm tra cấu hình các route hiện tại của ứng dụng.
Hiển Thị Middleware và Tên Các Nhóm Middleware
Mặc định, lệnh route:list
không hiển thị các middleware gán cho mỗi route. Để hiển thị các middleware và tên các nhóm middleware, bạn có thể sử dụng tùy chọn -v
:
php artisan route:list -v
Thêm -v
(verbose) vào lệnh sẽ hiển thị thêm các thông tin về các middleware áp dụng cho từng route. Nếu bạn muốn mở rộng thêm thông tin về các nhóm middleware, bạn có thể sử dụng -vv
:
php artisan route:list -vv
Tùy chọn -vv
cung cấp một cái nhìn chi tiết hơn về các nhóm middleware và các middleware cá nhân được gán cho các route, giúp bạn thấy rõ hơn cách các route được xử lý.
Hiển Thị Các Route Bắt Đầu Với Một URI Cụ Thể
Để chỉ hiển thị các route bắt đầu với một URI cụ thể, bạn có thể sử dụng tùy chọn --path
:
php artisan route:list --path=api
Thêm tùy chọn --path=api
sẽ lọc các route và chỉ hiển thị các route có URI bắt đầu bằng /api
. Đây là cách hữu ích để bạn chỉ xem các route liên quan đến API của bạn.
Ẩn Các Route Được Định Nghĩa Bởi Các Gói Thứ Ba
Để ẩn bất kỳ route nào được định nghĩa bởi các gói bên ngoài, bạn có thể sử dụng tùy chọn --except-vendor
:
php artisan route:list --except-vendor
Tùy chọn --except-vendor
sẽ loại bỏ các route đến từ các gói bên ngoài, giúp bạn tập trung vào các route được định nghĩa trong ứng dụng của bạn.
Chỉ Hiển Thị Các Route Được Định Nghĩa Bởi Các Gói Thứ Ba
Ngược lại, để chỉ hiển thị các route được định nghĩa bởi các gói bên ngoài, bạn có thể sử dụng tùy chọn --only-vendor
:
php artisan route:list --only-vendor
Tùy chọn --only-vendor
sẽ lọc các route và chỉ hiển thị các route đến từ các gói bên ngoài, giúp bạn xem xét các route của bên thứ ba mà không bị phân tâm bởi các route của ứng dụng của bạn.
Tóm Tắt Các Tùy Chọn Của route:list
php artisan route:list
: Hiển thị danh sách tất cả các route.php artisan route:list -v
: Hiển thị danh sách route kèm theo thông tin về middleware và nhóm middleware.php artisan route:list -vv
: Hiển thị thông tin chi tiết về middleware và nhóm middleware.php artisan route:list --path=api
: Hiển thị các route có URI bắt đầu bằng/api
.php artisan route:list --except-vendor
: Ẩn các route từ các gói bên ngoài.php artisan route:list --only-vendor
: Chỉ hiển thị các route từ các gói bên ngoài.
Tóm lại
Route là một phần không thể thiếu trong bất kỳ ứng dụng Laravel nào. Việc hiểu rõ và sử dụng thành thạo các route cơ bản sẽ giúp bạn xây dựng ứng dụng một cách hiệu quả và dễ dàng. Hy vọng bài viết này đã giúp bạn có cái nhìn tổng quan về hệ thống routing trong Laravel.
# Optional Parameters
Khi làm việc với các route trong Laravel, đôi khi bạn cần định nghĩa một tham số route mà không phải lúc nào cũng có mặt trong URI. Bạn có thể thực hiện điều này bằng cách đặt dấu ?
sau tên tham số và cung cấp một giá trị mặc định cho biến tương ứng trong route. Dưới đây là cách làm việc với tham số route tùy chọn, cùng với các ví dụ và giải thích chi tiết.
Route::get('/user/{name?}', function (?string $name = null) {
return $name;
});
Route::get('/user/{name?}', function (?string $name = 'John') {
return $name;
});
1. Ràng Buộc Định Dạng Tham Số Route
Dưới đây là tổng hợp các cách sử dụng ràng buộc biểu thức chính quy cho tham số route trong Laravel:
// Ràng buộc tham số phải chỉ chứa các ký tự chữ cái
Route::get('/user/{name}', function (string $name) {
// ...
})->where('name', '[A-Za-z]+');
// Ràng buộc tham số phải chỉ chứa các chữ số
Route::get('/user/{id}', function (string $id) {
// ...
})->where('id', '[0-9]+');
// Ràng buộc nhiều tham số với các biểu thức chính quy khác nhau
Route::get('/user/{id}/{name}', function (string $id, string $name) {
// ...
})->where(['id' => '[0-9]+', 'name' => '[a-z]+']);
// Sử dụng các phương thức tiện ích để thêm ràng buộc
Route::get('/user/{id}/{name}', function (string $id, string $name) {
// ...
})->whereNumber('id')->whereAlpha('name');
Route::get('/user/{name}', function (string $name) {
// ...
})->whereAlphaNumeric('name');
Route::get('/user/{id}', function (string $id) {
// ...
})->whereUuid('id');
Route::get('/user/{id}', function (string $id) {
//
})->whereUlid('id');
Route::get('/category/{category}', function (string $category) {
// ...
})->whereIn('category', ['movie', 'song', 'painting']);
// Ràng buộc toàn cục cho tham số route
public function boot(): void
{
Route::pattern('id', '[0-9]+');
}
// Cho phép ký tự '/' trong giá trị tham số
Route::get('/search/{search}', function (string $search) {
return $search;
})->where('search', '.*');
# Route Naming
Trong Laravel, bạn có thể đặt tên cho các route để tiện lợi hơn trong việc tạo URL hoặc chuyển hướng. Điều này giúp quản lý và tham chiếu đến các route dễ dàng hơn. Dưới đây là cách sử dụng route được đặt tên và các ví dụ cụ thể.
1. Đặt Tên Cho Route
Bạn có thể đặt tên cho một route bằng cách sử dụng phương thức name
sau khi định nghĩa route.
Ví Dụ
Route::get('/user/profile', function () {
// ...
})->name('profile');
Giải Thích
->name('profile')
: Đặt tênprofile
cho route/user/profile
.
2. Đặt Tên Cho Route Trong Controller
Bạn cũng có thể đặt tên cho các route trỏ tới các action trong controller:
Ví Dụ
Route::get('/user/profile', [UserProfileController::class, 'show'])->name('profile');
Giải Thích
[UserProfileController::class, 'show']
: Định nghĩa route trỏ tới phương thứcshow
trong controllerUserProfileController
.->name('profile')
: Đặt tênprofile
cho route này.
3. Tạo URL Từ Route Được Đặt Tên
Sau khi đã đặt tên cho một route, bạn có thể sử dụng tên của route để tạo URL hoặc chuyển hướng bằng các hàm trợ giúp của Laravel như route
và redirect
.
Tạo URL
$url = route('profile');
// Chuyển hướng
return redirect()->route('profile');
return to_route('profile');
Giải Thích
route('profile')
: Tạo URL tới route có tênprofile
.redirect()->route('profile')
: Chuyển hướng tới route có tênprofile
.to_route('profile')
: Một cách khác để chuyển hướng tới route có tênprofile
.
4. Truyền Tham Số Cho Route Được Đặt Tên
Nếu route có các tham số, bạn có thể truyền các tham số này như một mảng đối số thứ hai cho hàm route
.
Ví Dụ
Route::get('/user/{id}/profile', function (string $id) {
// ...
})->name('profile');
$url = route('profile', ['id' => 1]);
Giải Thích
['id' => 1]
: Tham sốid
được truyền vào URL.
5. Thêm Tham Số Vào Query String
Nếu bạn truyền thêm các tham số vào mảng, các cặp key/value này sẽ tự động được thêm vào query string của URL.
Ví Dụ
Route::get('/user/{id}/profile', function (string $id) {
// ...
})->name('profile');
$url = route('profile', ['id' => 1, 'photos' => 'yes']);
// Kết quả: /user/1/profile?photos=yes
Giải Thích
['id' => 1, 'photos' => 'yes']
: Tham sốphotos
được thêm vào query string của URL.
6. Thiết Lập Giá Trị Mặc Định Cho Tham Số URL
Bạn có thể thiết lập các giá trị mặc định cho tham số URL sử dụng phương thức URL::defaults
.
7. Kiểm Tra Route Hiện Tại
Bạn có thể kiểm tra xem request hiện tại có được định tuyến tới một route cụ thể hay không bằng phương thức named
trên một instance của Route. Điều này hữu ích khi làm việc với middleware.
Ví Dụ Trong Middleware
use Closure;
use Illuminate\Http\Request;
use Symfony\Component\HttpFoundation\Response;
public function handle(Request $request, Closure $next): Response
{
if ($request->route()->named('profile')) {
// ...
}
return $next($request);
}
Giải Thích
$request->route()->named('profile')
: Kiểm tra nếu route hiện tại có tênprofile
.
# Route Caching
Khi triển khai ứng dụng Laravel lên môi trường sản xuất, bạn nên tận dụng khả năng cache route của Laravel để tăng tốc độ xử lý. Việc sử dụng cache route sẽ giảm đáng kể thời gian cần thiết để đăng ký tất cả các route của ứng dụng. Dưới đây là cách tạo và quản lý cache route trong Laravel.
1. Tạo Route Cache
Để tạo cache cho các route, bạn sử dụng lệnh Artisan route:cache
:
php artisan route:cache
Giải Thích
- Lệnh
php artisan route:cache
: Tạo một file cache chứa tất cả các route của ứng dụng. Sau khi lệnh này được thực thi, file cache sẽ được tải lên mỗi khi có yêu cầu đến ứng dụng, giúp tăng tốc độ xử lý route.
2. Lưu Ý Khi Sử Dụng Route Cache
Sau khi chạy lệnh route:cache
, file cache sẽ được tải mỗi khi có yêu cầu đến ứng dụng. Tuy nhiên, nếu bạn thêm hoặc thay đổi bất kỳ route nào, bạn cần tạo lại cache mới. Do đó, bạn chỉ nên chạy lệnh route:cache
trong quá trình triển khai (deployment) của dự án.
3. Xóa Route Cache
Nếu bạn cần xóa cache route, bạn có thể sử dụng lệnh Artisan route:clear
:
php artisan route:clear
Giải Thích
- Lệnh
php artisan route:clear
: Xóa bỏ file cache route hiện tại. Điều này hữu ích khi bạn muốn cập nhật route mà không cần tạo cache mới ngay lập tức.
Quy Trình Sử Dụng Route Cache Trong Deployment
- Triển khai ứng dụng lên môi trường sản xuất.
- Chạy lệnh
php artisan route:cache
để tạo file cache cho route. - Khi cần cập nhật hoặc thêm route mới, hãy chạy lệnh
php artisan route:clear
để xóa cache hiện tại và sau đó tạo lại cache bằng lệnhphp artisan route:cache
.
Ví Dụ Quy Trình Deployment
# Bước 1: Triển khai ứng dụng
# (Thực hiện các bước triển khai thông thường như kéo code mới, cài đặt dependency, v.v.)
# Bước 2: Tạo route cache
php artisan route:cache
# Sau khi thêm hoặc thay đổi route
# Bước 3: Xóa route cache hiện tại
php artisan route:clear
# Bước 4: Tạo lại route cache mới
php artisan route:cache
Tóm Tắt
Việc sử dụng cache route trong Laravel giúp cải thiện hiệu suất của ứng dụng khi triển khai lên môi trường sản xuất. Tuy nhiên, bạn cần nhớ rằng mỗi khi thay đổi hoặc thêm mới route, bạn phải xóa cache cũ và tạo lại cache mới. Điều này đảm bảo rằng ứng dụng của bạn luôn sử dụng các route mới nhất mà bạn đã định nghĩa.
# Tạo cache cho route php artisan route:cache # Xóa cache route hiện tại php artisan route:clear
Bằng cách tuân thủ quy trình trên, bạn sẽ có thể tận dụng tối đa hiệu quả của tính năng route cache trong Laravel, giúp ứng dụng của bạn hoạt động nhanh hơn và hiệu quả hơn.
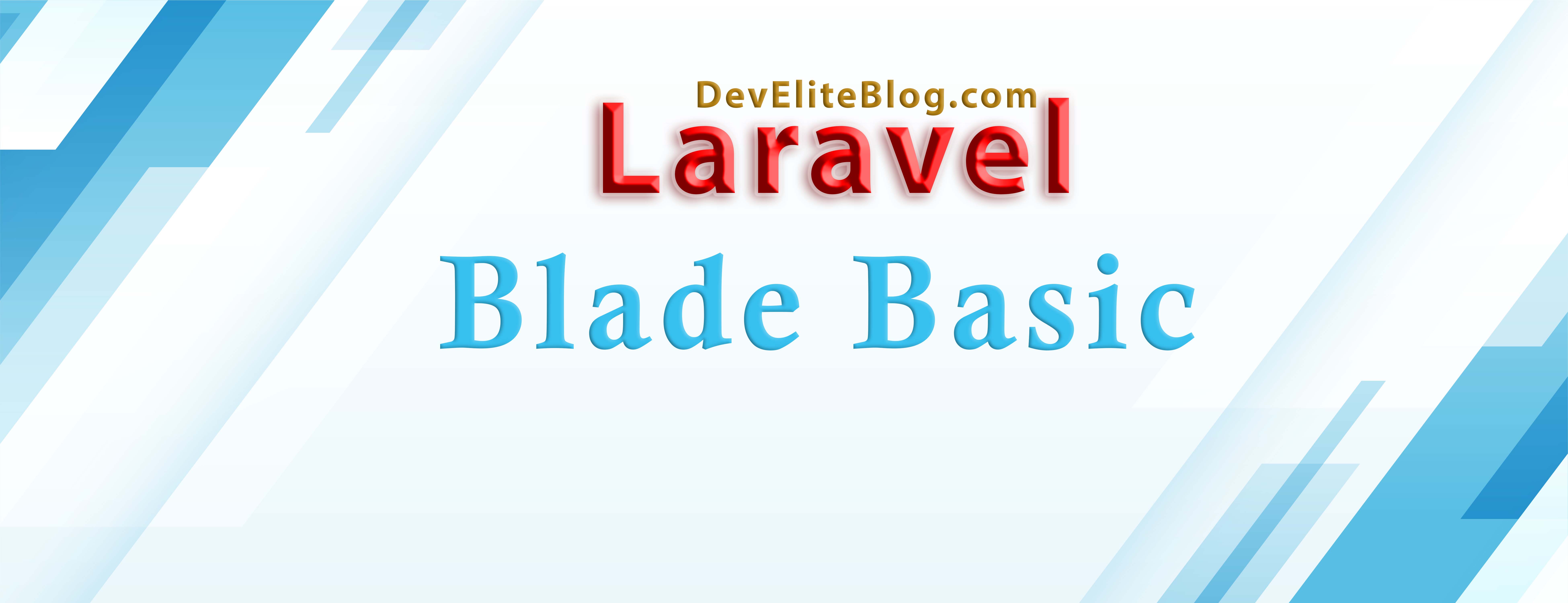
Blade Basics
Author: | ADMIN |
---|
# Blade Basics
Blade là một công cụ tạo template (template engine) mạnh mẽ được tích hợp sẵn trong Laravel. Nó cho phép bạn sử dụng các cấu trúc điều khiển như if, for, while,... ngay trong các file template của bạn. Dưới đây là một số khái niệm cơ bản và ví dụ minh họa để bạn có thể bắt đầu sử dụng Blade.
1. Tạo Và Sử Dụng File Blade
Các file Blade có đuôi mở rộng là .blade.php
. Ví dụ, bạn có thể tạo một file Blade cho trang chủ của ứng dụng như sau:
<!-- resources/views/home.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Home Page</title>
</head>
<body>
<h1>Welcome to My Laravel Application</h1>
</body>
</html>
Để trả về view này từ một route, bạn có thể sử dụng phương thức view
của Laravel:
Route::get('/', function () {
return view('home');
});
2. Các Cấu Trúc Điều Khiển
If Statements
Blade hỗ trợ các cấu trúc điều khiển như if
, elseif
, else
, và endif
:
<!-- resources/views/home.blade.php -->
@if ($name == 'John')
<p>Hello, John!</p>
@elseif ($name == 'Jane')
<p>Hello, Jane!</p>
@else
<p>Hello, Stranger!</p>
@endif
Loops
Blade cũng hỗ trợ các cấu trúc lặp như for
, foreach
, forelse
, và while
:
<!-- resources/views/home.blade.php -->
@foreach ($users as $user)
<p>This is user {{ $user->name }}</p>
@endforeach
3. Echoing Data
Blade cung cấp cú pháp đơn giản để hiển thị dữ liệu từ các biến PHP:
<!-- resources/views/home.blade.php -->
<p>{{ $name }}</p>
<p>{{ $age }}</p>
Bạn cũng có thể sử dụng hàm @php
để nhúng mã PHP trực tiếp vào file Blade:
<!-- resources/views/home.blade.php -->
@php
$name = 'John';
@endphp
<p>{{ $name }}</p>
4. Escape HTML
Blade sẽ tự động escape các biến để bảo vệ ứng dụng của bạn khỏi các lỗ hổng XSS. Nếu bạn muốn hiển thị dữ liệu mà không escape, bạn có thể sử dụng cú pháp sau:
<!-- resources/views/home.blade.php -->
{!! $unescapedData !!}
5. Kế Thừa Layouts
Blade cung cấp cơ chế kế thừa layout giúp bạn tạo ra các template có cấu trúc lặp lại một cách dễ dàng.
Tạo Layout
<!-- resources/views/layouts/app.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>@yield('title')</title>
</head>
<body>
<div class="container">
@yield('content')
</div>
</body>
</html>
Sử Dụng Layout
<!-- resources/views/home.blade.php -->
@extends('layouts.app')
@section('title', 'Home Page')
@section('content')
<h1>Welcome to My Laravel Application</h1>
@endsection
6. Bao Gồm Các View Con (Including Sub-Views)
Bạn có thể chia nhỏ view thành các phần nhỏ hơn và bao gồm chúng vào view chính:
<!-- resources/views/includes/header.blade.php -->
<header>
<h1>Header Content</h1>
</header>
<!-- resources/views/home.blade.php -->
@include('includes.header')
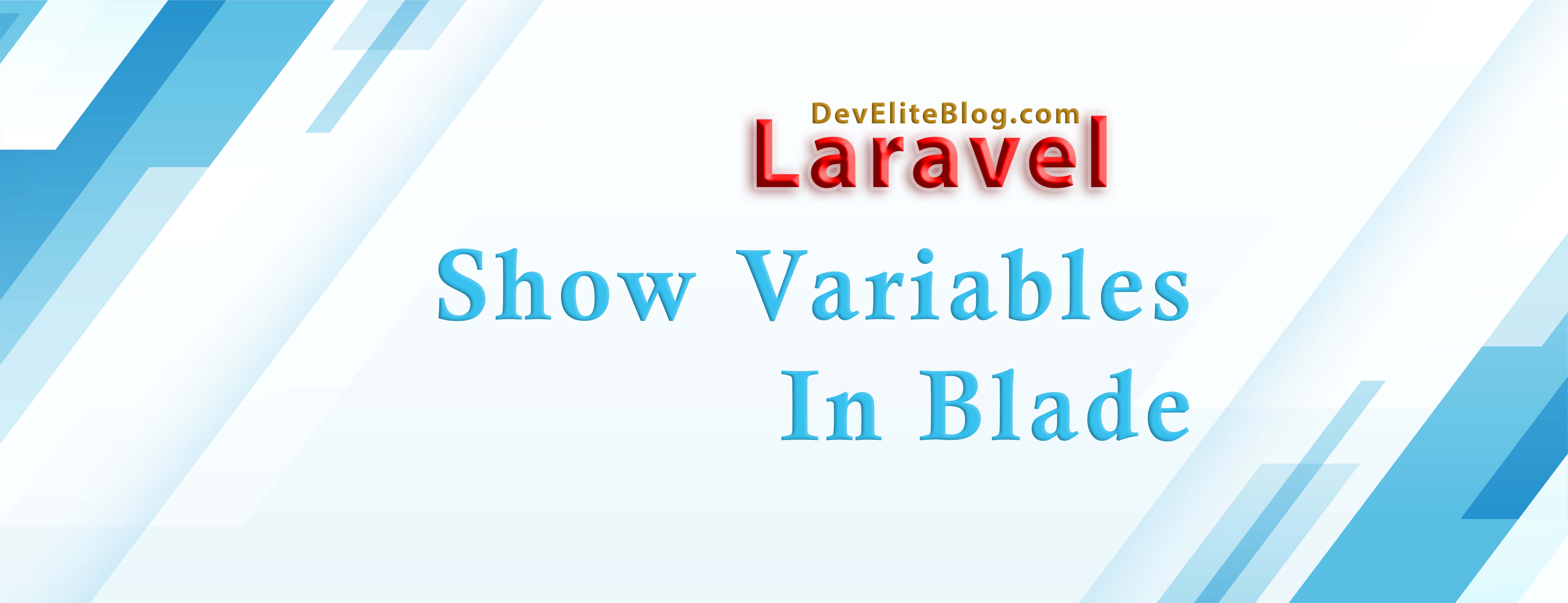
Hiển thị giá trị trong Blade
Author: | ADMIN |
---|
# Hiển Thị Biến Trong Blade
Trong Laravel Blade, việc hiển thị biến rất đơn giản và trực quan. Blade cung cấp cú pháp dễ đọc và sử dụng để nhúng các biến PHP vào trong HTML.
Cú Pháp Cơ Bản
Sử Dụng Cặp Dấu Ngoặc Nhanh {{ }}
Để hiển thị giá trị của một biến trong Blade, bạn chỉ cần sử dụng cặp dấu ngoặc nhanh {{ }}
.
Ví Dụ
Giả sử bạn có một biến $name
được truyền từ controller đến view:
public function show()
{
$name = 'John Doe';
return view('greeting', compact('name'));
}
Trong file view greeting.blade.php
, bạn có thể hiển thị giá trị của biến $name
như sau:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Greeting Page</title>
</head>
<body>
<h1>Hello, {{ $name }}!</h1>
</body>
</html>
Escape HTML Đầu Vào
Khi sử dụng cặp dấu ngoặc nhanh {{ }}
, Blade sẽ tự động escape (chuyển đổi các ký tự đặc biệt thành các thực thể HTML) để bảo vệ chống lại các cuộc tấn công XSS.
Ví Dụ
<?php $name = '<script>alert("XSS")</script>'; ?>
<h1>Hello, {{ $name }}!</h1>
Kết quả hiển thị sẽ là:
<h1>Hello, <script>alert("XSS")</script>!</h1>
Hiển Thị Biến Mà Không Escape HTML
Nếu bạn muốn hiển thị giá trị của biến mà không escape HTML, bạn có thể sử dụng cú pháp {!! !!}
.
<?php $name = '<strong>John Doe</strong>'; ?>
<h1>Hello, {!! $name !!}!</h1>
Kết quả hiển thị sẽ là:
<h1>Hello, <strong>John Doe</strong>!</h1>
Hiển Thị Các Giá Trị Mặc Định
Bạn có thể hiển thị các giá trị mặc định khi biến không tồn tại hoặc rỗng bằng cách sử dụng toán tử null coalescing ??
.
Ví Dụ
<h1>Hello, {{ $name ?? 'Guest' }}!</h1>
Nếu biến $name
không tồn tại hoặc có giá trị là null, chuỗi 'Guest'
sẽ được hiển thị.
Hiển Thị Mảng và Đối Tượng
Bạn có thể truy cập và hiển thị các phần tử của mảng hoặc thuộc tính của đối tượng một cách dễ dàng.
Ví Dụ Mảng
<?php $user = ['name' => 'John Doe', 'email' => 'john@example.com']; ?>
<h1>Name: {{ $user['name'] }}</h1>
<p>Email: {{ $user['email'] }}</p>
Ví Dụ Đối Tượng
<?php $user = (object) ['name' => 'John Doe', 'email' => 'john@example.com']; ?>
<h1>Name: {{ $user->name }}</h1>
<p>Email: {{ $user->email }}</p>
Tóm Tắt
- Cú pháp cơ bản: Sử dụng
{{ $variable }}
để hiển thị giá trị của biến và tự động escape HTML.- Không escape HTML: Sử dụng
{!! $variable !!}
để hiển thị giá trị của biến mà không escape HTML.- Giá trị mặc định: Sử dụng
{{ $variable ?? 'default' }}
để hiển thị giá trị mặc định khi biến không tồn tại hoặc rỗng.- Mảng và đối tượng: Truy cập và hiển thị các phần tử của mảng hoặc thuộc tính của đối tượng bằng cú pháp
{{ $array['key'] }}
và{{ $object->property }}
.Việc sử dụng các cú pháp này giúp bạn dễ dàng và an toàn khi hiển thị dữ liệu trong các view Blade của Laravel.
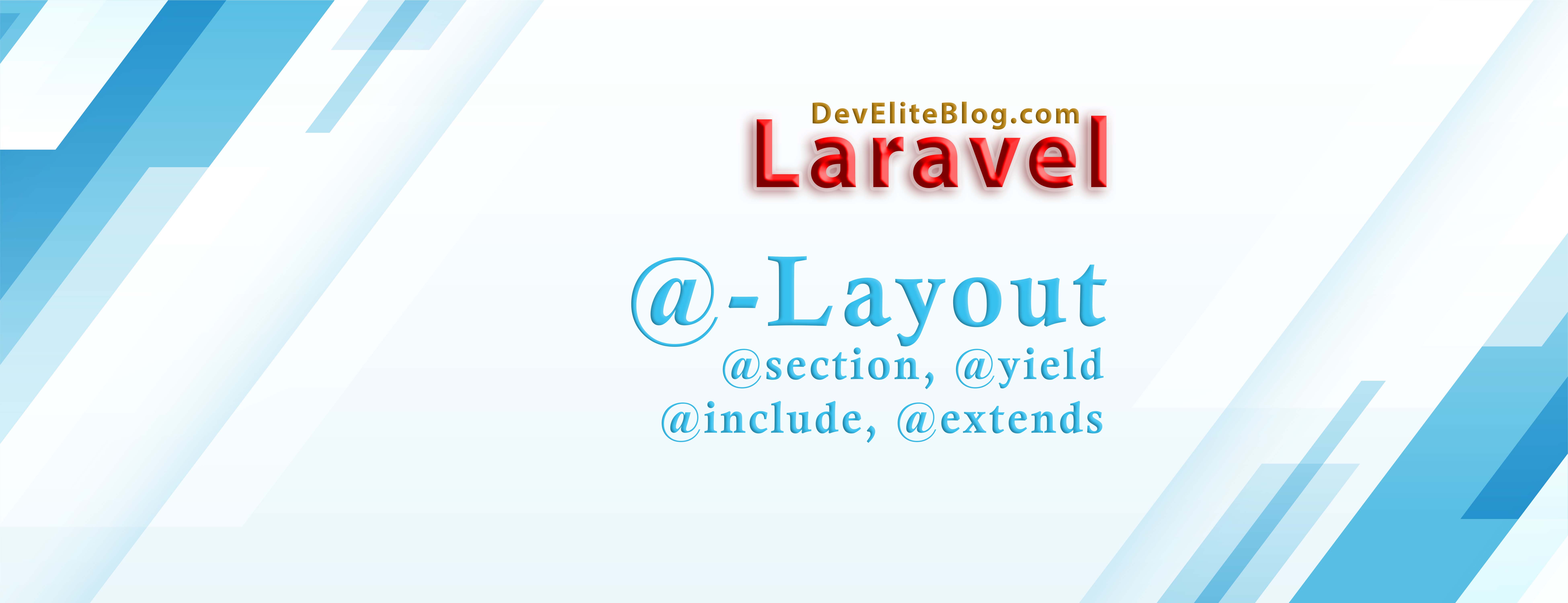
Layout: @include, @extends, @section, @yield
Author: | ADMIN |
---|
# @include, @extends, @section, và @yield
Laravel Blade cung cấp các từ khóa @include
, @extends
, @section
, và @yield
để giúp bạn tái sử dụng và tổ chức mã HTML một cách hiệu quả. Dưới đây là cách sử dụng từng từ khóa này với các ví dụ cụ thể.
@include
@include
cho phép bạn nhúng một view Blade khác vào trong view hiện tại. Đây là cách tuyệt vời để tái sử dụng các phần tử HTML như header, footer, hoặc sidebar.
Ví Dụ
Giả sử bạn có một view header.blade.php
:
<!-- resources/views/header.blade.php -->
<header>
<h1>My Website Header</h1>
</header>
Bạn có thể nhúng view này vào view chính của bạn như sau:
<!-- resources/views/welcome.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Welcome</title>
</head>
<body>
@include('header')
<div class="content">
<p>Welcome to the homepage!</p>
</div>
</body>
</html>
@extends
@extends
được sử dụng để chỉ định layout mà view hiện tại sẽ kế thừa. Layout này thường chứa các cấu trúc HTML cơ bản mà các view khác có thể sử dụng lại.
Ví Dụ
Giả sử bạn có một layout app.blade.php
:
<!-- resources/views/layouts/app.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>@yield('title')</title>
</head>
<body>
@include('header')
<div class="content">
@yield('content')
</div>
@include('footer')
</body>
</html>
Trong view cụ thể của bạn, bạn có thể kế thừa layout này như sau:
<!-- resources/views/home.blade.php -->
@extends('layouts.app')
@section('title', 'Home Page')
@section('content')
<h2>Welcome to the Home Page</h2>
<p>This is the home page content.</p>
@endsection
@section và @yield
@section
được sử dụng để xác định nội dung mà view con sẽ cung cấp cho các vùng nội dung được định nghĩa trong layout bằng @yield
.
Ví Dụ
Trong layout app.blade.php
, bạn có thể có các vùng nội dung:
<!-- resources/views/layouts/app.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>@yield('title')</title>
</head>
<body>
@include('header')
<div class="content">
@yield('content')
</div>
@include('footer')
</body>
</html>
Trong view con home.blade.php
, bạn có thể định nghĩa các phần nội dung cho các vùng đó:
<!-- resources/views/home.blade.php -->
@extends('layouts.app')
@section('title', 'Home Page')
@section('content')
<h2>Welcome to the Home Page</h2>
<p>This is the home page content.</p>
@endsection
Tóm Tắt
- @include: Nhúng một view Blade khác vào view hiện tại.
- @extends: Kế thừa layout từ một view khác.
- @section: Định nghĩa một phần nội dung trong view con.
- @yield: Đánh dấu vị trí mà nội dung của view con sẽ được chèn vào trong layout.
Việc sử dụng @include
, @extends
, @section
, và @yield
giúp bạn tổ chức mã nguồn của mình một cách rõ ràng và tái sử dụng được các phần tử giao diện, làm cho việc phát triển và bảo trì ứng dụng trở nên dễ dàng hơn.
Bài viết khác
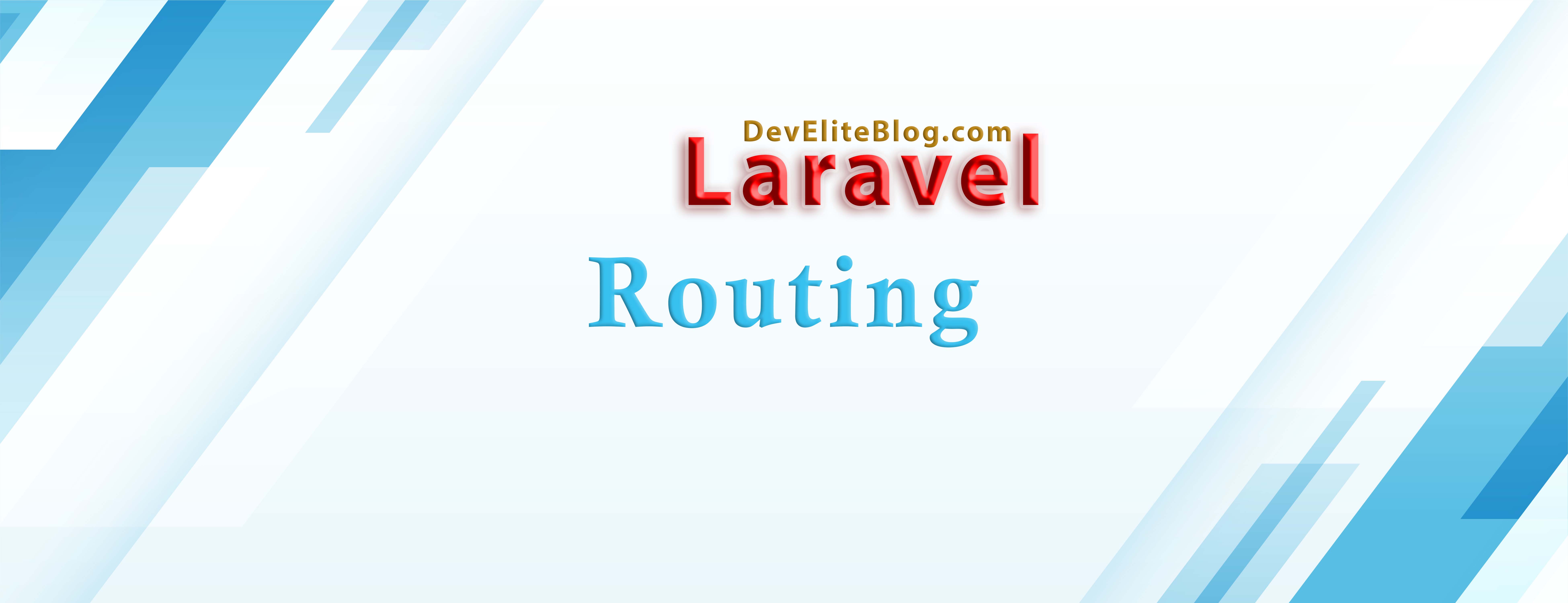
Routing
Author: | ADMIN |
---|
# Basic Routing
1. Khái niệm về Route
Route trong Laravel là cách bạn định nghĩa URL cho ứng dụng của mình. Route giúp bạn chỉ định URL nào sẽ gọi đến Controller nào hoặc thực hiện hành động gì.
2. Định nghĩa Route Cơ Bản
Tất cả các route của Laravel được định nghĩa trong các file nằm trong thư mục routes
. Các file này được tự động tải bởi App\Providers\RouteServiceProvider
của ứng dụng của bạn. Có bốn file route chính:
web.php
: Định nghĩa các route cho web application.api.php
: Định nghĩa các route cho API.console.php
: Định nghĩa các route cho console commands.channels.php
: Định nghĩa các route cho event broadcasting channels.
Định nghĩa route trong web.php
Dưới đây là một ví dụ đơn giản về cách định nghĩa một route trong file web.php
:
Route::get('/', function () {
return view('welcome');
});
Trong ví dụ trên:
Route::get('/')
: Định nghĩa một route sử dụng phương thức GET cho URL/
.function () { return view('welcome'); }
: Định nghĩa hành động sẽ thực hiện khi người dùng truy cập vào URL/
. Ở đây, Laravel sẽ trả về viewwelcome
.
3. Các Phương Thức Route
Laravel hỗ trợ nhiều phương thức HTTP khác nhau, bao gồm:
GET
: Dùng để truy xuất dữ liệu từ server.POST
: Dùng để gửi dữ liệu lên server.PUT
: Dùng để cập nhật dữ liệu trên server.PATCH
: Dùng để cập nhật một phần tài nguyên trên server. Tương tự nhưPUT
nhưng chỉ cập nhật các phần cụ thể của tài nguyên.DELETE
: Dùng để xóa dữ liệu từ server.OPTIONS
: Dùng để truy vấn các phương thức HTTP mà server hỗ trợ cho một URL cụ thể. Thường được sử dụng trong các ứng dụng RESTful API để kiểm tra các tùy chọn giao tiếp.
Ví dụ về các phương thức khác nhau:
Route::get('/products', function () {
return 'Get all products';
});
Route::post('/products', function () {
return 'Create a new product';
});
Route::put('/products/{id}', function ($id) {
return 'Update the product with ID ' . $id;
});
Route::patch('/products/{id}', function ($id) {
return 'Partially update the product with ID ' . $id;
});
Route::delete('/products/{id}', function ($id) {
return 'Delete the product with ID ' . $id;
});
Route::options('/products', function () {
return response()->json(['GET', 'POST', 'PUT', 'PATCH', 'DELETE']);
});
4. Route với Tham Số
Bạn có thể định nghĩa route có tham số như sau:
Route::get('/user/{id}', function ($id) {
return 'User '.$id;
});
Trong ví dụ này, {id}
là một tham số động. Khi người dùng truy cập vào URL user/1
, Laravel sẽ gán giá trị 1
vào biến $id
và trả về User 1
.
5. Route Groups
Bạn có thể nhóm các route lại với nhau bằng cách sử dụng Route::group
. Điều này giúp bạn dễ dàng áp dụng middleware hoặc tiền tố URL cho một nhóm route.
Route::group(['prefix' => 'admin'], function () {
Route::get('/users', function () {
// Matches The "/admin/users" URL
});
Route::get('/settings', function () {
// Matches The "/admin/settings" URL
});
});
Trong ví dụ trên, tất cả các route trong nhóm này sẽ có tiền tố admin
.
6. Middleware
Middleware là các lớp trung gian mà HTTP request phải đi qua trước khi đến Controller. Bạn có thể áp dụng middleware cho route như sau:
Route::get('/profile', function () {
// Only authenticated users may enter...
})->middleware('auth');
Trong ví dụ này, middleware auth
sẽ kiểm tra xem người dùng có được xác thực hay không trước khi cho phép truy cập vào route /profile
.
Laravel cung cấp hai nhóm middleware chính cho các file route:
- web middleware group: Được áp dụng cho các route trong
routes/web.php
. Middleware nhóm này bao gồm các tính năng như:- Session state
- CSRF protection
- Cookie encryption
- ...
- api middleware group: Được áp dụng cho các route trong
routes/api.php
. Middleware nhóm này bao gồm các tính năng như:- Stateless (không trạng thái)
- Token-based authentication
- Rate limiting
- ...
7. CSRF Protection
Hãy nhớ rằng, bất kỳ biểu mẫu HTML nào trỏ tới các route sử dụng phương thức POST, PUT, PATCH, hoặc DELETE được định nghĩa trong file route web cần phải bao gồm trường token CSRF. Nếu không, yêu cầu sẽ bị từ chối. Bạn có thể đọc thêm về bảo vệ CSRF trong tài liệu CSRF:
<form method="POST" action="/profile">
@csrf
<!-- Các trường input khác -->
<button type="submit">Submit</button>
</form>
Việc bảo vệ CSRF rất quan trọng để ngăn chặn các tấn công giả mạo yêu cầu từ trang chéo, đảm bảo rằng các yêu cầu tới server đến từ nguồn đáng tin cậy.
8. Redirect Routes
Nếu bạn định nghĩa một route để chuyển hướng đến một URI khác, bạn có thể sử dụng phương thức Route::redirect
. Phương thức này cung cấp một cách tắt thuận tiện để bạn không phải định nghĩa một route hoặc controller đầy đủ cho việc thực hiện chuyển hướng đơn giản.
Ví dụ:
Route::redirect('/here', '/there');
Mặc định, Route::redirect
sẽ trả về mã trạng thái 302. Mã trạng thái 302 cho biết rằng tài nguyên đã được di chuyển tạm thời đến một vị trí mới.
Tuỳ Chỉnh Mã Trạng Thái
Bạn có thể tuỳ chỉnh mã trạng thái bằng cách sử dụng tham số thứ ba tùy chọn:
Route::redirect('/here', '/there', 301);
Trong ví dụ này, mã trạng thái 301 được sử dụng để chỉ ra rằng tài nguyên đã được di chuyển vĩnh viễn đến một vị trí mới.
Sử Dụng Route::permanentRedirect
Hoặc, bạn có thể sử dụng phương thức Route::permanentRedirect
để trả về mã trạng thái 301:
Route::permanentRedirect('/here', '/there');
9. The Route List
Lệnh Artisan route:list
là một công cụ mạnh mẽ giúp bạn dễ dàng xem tất cả các route đã được định nghĩa trong ứng dụng của bạn. Dưới đây là các cách sử dụng lệnh route:list
cùng với giải thích chi tiết:
Hiển Thị Danh Sách Các Route
Để xem tất cả các route được định nghĩa trong ứng dụng của bạn, bạn có thể sử dụng lệnh:
php artisan route:list
Lệnh này hiển thị một bảng tổng quan của tất cả các route trong ứng dụng, bao gồm các thông tin như phương thức HTTP, URI, tên route, và hành động (action) của route đó. Đây là cách nhanh chóng để bạn kiểm tra cấu hình các route hiện tại của ứng dụng.
Hiển Thị Middleware và Tên Các Nhóm Middleware
Mặc định, lệnh route:list
không hiển thị các middleware gán cho mỗi route. Để hiển thị các middleware và tên các nhóm middleware, bạn có thể sử dụng tùy chọn -v
:
php artisan route:list -v
Thêm -v
(verbose) vào lệnh sẽ hiển thị thêm các thông tin về các middleware áp dụng cho từng route. Nếu bạn muốn mở rộng thêm thông tin về các nhóm middleware, bạn có thể sử dụng -vv
:
php artisan route:list -vv
Tùy chọn -vv
cung cấp một cái nhìn chi tiết hơn về các nhóm middleware và các middleware cá nhân được gán cho các route, giúp bạn thấy rõ hơn cách các route được xử lý.
Hiển Thị Các Route Bắt Đầu Với Một URI Cụ Thể
Để chỉ hiển thị các route bắt đầu với một URI cụ thể, bạn có thể sử dụng tùy chọn --path
:
php artisan route:list --path=api
Thêm tùy chọn --path=api
sẽ lọc các route và chỉ hiển thị các route có URI bắt đầu bằng /api
. Đây là cách hữu ích để bạn chỉ xem các route liên quan đến API của bạn.
Ẩn Các Route Được Định Nghĩa Bởi Các Gói Thứ Ba
Để ẩn bất kỳ route nào được định nghĩa bởi các gói bên ngoài, bạn có thể sử dụng tùy chọn --except-vendor
:
php artisan route:list --except-vendor
Tùy chọn --except-vendor
sẽ loại bỏ các route đến từ các gói bên ngoài, giúp bạn tập trung vào các route được định nghĩa trong ứng dụng của bạn.
Chỉ Hiển Thị Các Route Được Định Nghĩa Bởi Các Gói Thứ Ba
Ngược lại, để chỉ hiển thị các route được định nghĩa bởi các gói bên ngoài, bạn có thể sử dụng tùy chọn --only-vendor
:
php artisan route:list --only-vendor
Tùy chọn --only-vendor
sẽ lọc các route và chỉ hiển thị các route đến từ các gói bên ngoài, giúp bạn xem xét các route của bên thứ ba mà không bị phân tâm bởi các route của ứng dụng của bạn.
Tóm Tắt Các Tùy Chọn Của route:list
php artisan route:list
: Hiển thị danh sách tất cả các route.php artisan route:list -v
: Hiển thị danh sách route kèm theo thông tin về middleware và nhóm middleware.php artisan route:list -vv
: Hiển thị thông tin chi tiết về middleware và nhóm middleware.php artisan route:list --path=api
: Hiển thị các route có URI bắt đầu bằng/api
.php artisan route:list --except-vendor
: Ẩn các route từ các gói bên ngoài.php artisan route:list --only-vendor
: Chỉ hiển thị các route từ các gói bên ngoài.
Tóm lại
Route là một phần không thể thiếu trong bất kỳ ứng dụng Laravel nào. Việc hiểu rõ và sử dụng thành thạo các route cơ bản sẽ giúp bạn xây dựng ứng dụng một cách hiệu quả và dễ dàng. Hy vọng bài viết này đã giúp bạn có cái nhìn tổng quan về hệ thống routing trong Laravel.
# Optional Parameters
Khi làm việc với các route trong Laravel, đôi khi bạn cần định nghĩa một tham số route mà không phải lúc nào cũng có mặt trong URI. Bạn có thể thực hiện điều này bằng cách đặt dấu ?
sau tên tham số và cung cấp một giá trị mặc định cho biến tương ứng trong route. Dưới đây là cách làm việc với tham số route tùy chọn, cùng với các ví dụ và giải thích chi tiết.
Route::get('/user/{name?}', function (?string $name = null) {
return $name;
});
Route::get('/user/{name?}', function (?string $name = 'John') {
return $name;
});
1. Ràng Buộc Định Dạng Tham Số Route
Dưới đây là tổng hợp các cách sử dụng ràng buộc biểu thức chính quy cho tham số route trong Laravel:
// Ràng buộc tham số phải chỉ chứa các ký tự chữ cái
Route::get('/user/{name}', function (string $name) {
// ...
})->where('name', '[A-Za-z]+');
// Ràng buộc tham số phải chỉ chứa các chữ số
Route::get('/user/{id}', function (string $id) {
// ...
})->where('id', '[0-9]+');
// Ràng buộc nhiều tham số với các biểu thức chính quy khác nhau
Route::get('/user/{id}/{name}', function (string $id, string $name) {
// ...
})->where(['id' => '[0-9]+', 'name' => '[a-z]+']);
// Sử dụng các phương thức tiện ích để thêm ràng buộc
Route::get('/user/{id}/{name}', function (string $id, string $name) {
// ...
})->whereNumber('id')->whereAlpha('name');
Route::get('/user/{name}', function (string $name) {
// ...
})->whereAlphaNumeric('name');
Route::get('/user/{id}', function (string $id) {
// ...
})->whereUuid('id');
Route::get('/user/{id}', function (string $id) {
//
})->whereUlid('id');
Route::get('/category/{category}', function (string $category) {
// ...
})->whereIn('category', ['movie', 'song', 'painting']);
// Ràng buộc toàn cục cho tham số route
public function boot(): void
{
Route::pattern('id', '[0-9]+');
}
// Cho phép ký tự '/' trong giá trị tham số
Route::get('/search/{search}', function (string $search) {
return $search;
})->where('search', '.*');
# Route Naming
Trong Laravel, bạn có thể đặt tên cho các route để tiện lợi hơn trong việc tạo URL hoặc chuyển hướng. Điều này giúp quản lý và tham chiếu đến các route dễ dàng hơn. Dưới đây là cách sử dụng route được đặt tên và các ví dụ cụ thể.
1. Đặt Tên Cho Route
Bạn có thể đặt tên cho một route bằng cách sử dụng phương thức name
sau khi định nghĩa route.
Ví Dụ
Route::get('/user/profile', function () {
// ...
})->name('profile');
Giải Thích
->name('profile')
: Đặt tênprofile
cho route/user/profile
.
2. Đặt Tên Cho Route Trong Controller
Bạn cũng có thể đặt tên cho các route trỏ tới các action trong controller:
Ví Dụ
Route::get('/user/profile', [UserProfileController::class, 'show'])->name('profile');
Giải Thích
[UserProfileController::class, 'show']
: Định nghĩa route trỏ tới phương thứcshow
trong controllerUserProfileController
.->name('profile')
: Đặt tênprofile
cho route này.
3. Tạo URL Từ Route Được Đặt Tên
Sau khi đã đặt tên cho một route, bạn có thể sử dụng tên của route để tạo URL hoặc chuyển hướng bằng các hàm trợ giúp của Laravel như route
và redirect
.
Tạo URL
$url = route('profile');
// Chuyển hướng
return redirect()->route('profile');
return to_route('profile');
Giải Thích
route('profile')
: Tạo URL tới route có tênprofile
.redirect()->route('profile')
: Chuyển hướng tới route có tênprofile
.to_route('profile')
: Một cách khác để chuyển hướng tới route có tênprofile
.
4. Truyền Tham Số Cho Route Được Đặt Tên
Nếu route có các tham số, bạn có thể truyền các tham số này như một mảng đối số thứ hai cho hàm route
.
Ví Dụ
Route::get('/user/{id}/profile', function (string $id) {
// ...
})->name('profile');
$url = route('profile', ['id' => 1]);
Giải Thích
['id' => 1]
: Tham sốid
được truyền vào URL.
5. Thêm Tham Số Vào Query String
Nếu bạn truyền thêm các tham số vào mảng, các cặp key/value này sẽ tự động được thêm vào query string của URL.
Ví Dụ
Route::get('/user/{id}/profile', function (string $id) {
// ...
})->name('profile');
$url = route('profile', ['id' => 1, 'photos' => 'yes']);
// Kết quả: /user/1/profile?photos=yes
Giải Thích
['id' => 1, 'photos' => 'yes']
: Tham sốphotos
được thêm vào query string của URL.
6. Thiết Lập Giá Trị Mặc Định Cho Tham Số URL
Bạn có thể thiết lập các giá trị mặc định cho tham số URL sử dụng phương thức URL::defaults
.
7. Kiểm Tra Route Hiện Tại
Bạn có thể kiểm tra xem request hiện tại có được định tuyến tới một route cụ thể hay không bằng phương thức named
trên một instance của Route. Điều này hữu ích khi làm việc với middleware.
Ví Dụ Trong Middleware
use Closure;
use Illuminate\Http\Request;
use Symfony\Component\HttpFoundation\Response;
public function handle(Request $request, Closure $next): Response
{
if ($request->route()->named('profile')) {
// ...
}
return $next($request);
}
Giải Thích
$request->route()->named('profile')
: Kiểm tra nếu route hiện tại có tênprofile
.
# Route Caching
Khi triển khai ứng dụng Laravel lên môi trường sản xuất, bạn nên tận dụng khả năng cache route của Laravel để tăng tốc độ xử lý. Việc sử dụng cache route sẽ giảm đáng kể thời gian cần thiết để đăng ký tất cả các route của ứng dụng. Dưới đây là cách tạo và quản lý cache route trong Laravel.
1. Tạo Route Cache
Để tạo cache cho các route, bạn sử dụng lệnh Artisan route:cache
:
php artisan route:cache
Giải Thích
- Lệnh
php artisan route:cache
: Tạo một file cache chứa tất cả các route của ứng dụng. Sau khi lệnh này được thực thi, file cache sẽ được tải lên mỗi khi có yêu cầu đến ứng dụng, giúp tăng tốc độ xử lý route.
2. Lưu Ý Khi Sử Dụng Route Cache
Sau khi chạy lệnh route:cache
, file cache sẽ được tải mỗi khi có yêu cầu đến ứng dụng. Tuy nhiên, nếu bạn thêm hoặc thay đổi bất kỳ route nào, bạn cần tạo lại cache mới. Do đó, bạn chỉ nên chạy lệnh route:cache
trong quá trình triển khai (deployment) của dự án.
3. Xóa Route Cache
Nếu bạn cần xóa cache route, bạn có thể sử dụng lệnh Artisan route:clear
:
php artisan route:clear
Giải Thích
- Lệnh
php artisan route:clear
: Xóa bỏ file cache route hiện tại. Điều này hữu ích khi bạn muốn cập nhật route mà không cần tạo cache mới ngay lập tức.
Quy Trình Sử Dụng Route Cache Trong Deployment
- Triển khai ứng dụng lên môi trường sản xuất.
- Chạy lệnh
php artisan route:cache
để tạo file cache cho route. - Khi cần cập nhật hoặc thêm route mới, hãy chạy lệnh
php artisan route:clear
để xóa cache hiện tại và sau đó tạo lại cache bằng lệnhphp artisan route:cache
.
Ví Dụ Quy Trình Deployment
# Bước 1: Triển khai ứng dụng
# (Thực hiện các bước triển khai thông thường như kéo code mới, cài đặt dependency, v.v.)
# Bước 2: Tạo route cache
php artisan route:cache
# Sau khi thêm hoặc thay đổi route
# Bước 3: Xóa route cache hiện tại
php artisan route:clear
# Bước 4: Tạo lại route cache mới
php artisan route:cache
Tóm Tắt
Việc sử dụng cache route trong Laravel giúp cải thiện hiệu suất của ứng dụng khi triển khai lên môi trường sản xuất. Tuy nhiên, bạn cần nhớ rằng mỗi khi thay đổi hoặc thêm mới route, bạn phải xóa cache cũ và tạo lại cache mới. Điều này đảm bảo rằng ứng dụng của bạn luôn sử dụng các route mới nhất mà bạn đã định nghĩa.
# Tạo cache cho route php artisan route:cache # Xóa cache route hiện tại php artisan route:clear
Bằng cách tuân thủ quy trình trên, bạn sẽ có thể tận dụng tối đa hiệu quả của tính năng route cache trong Laravel, giúp ứng dụng của bạn hoạt động nhanh hơn và hiệu quả hơn.
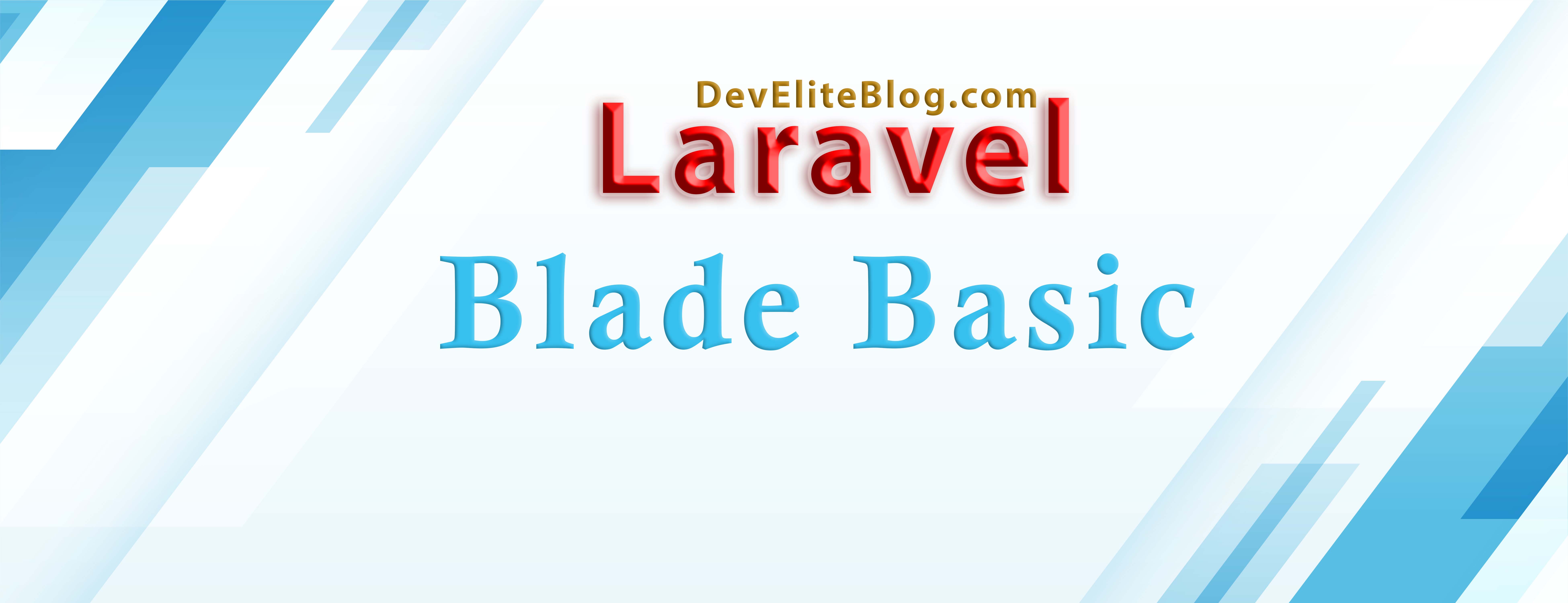
Blade Basics
Author: | ADMIN |
---|
# Blade Basics
Blade là một công cụ tạo template (template engine) mạnh mẽ được tích hợp sẵn trong Laravel. Nó cho phép bạn sử dụng các cấu trúc điều khiển như if, for, while,... ngay trong các file template của bạn. Dưới đây là một số khái niệm cơ bản và ví dụ minh họa để bạn có thể bắt đầu sử dụng Blade.
1. Tạo Và Sử Dụng File Blade
Các file Blade có đuôi mở rộng là .blade.php
. Ví dụ, bạn có thể tạo một file Blade cho trang chủ của ứng dụng như sau:
<!-- resources/views/home.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Home Page</title>
</head>
<body>
<h1>Welcome to My Laravel Application</h1>
</body>
</html>
Để trả về view này từ một route, bạn có thể sử dụng phương thức view
của Laravel:
Route::get('/', function () {
return view('home');
});
2. Các Cấu Trúc Điều Khiển
If Statements
Blade hỗ trợ các cấu trúc điều khiển như if
, elseif
, else
, và endif
:
<!-- resources/views/home.blade.php -->
@if ($name == 'John')
<p>Hello, John!</p>
@elseif ($name == 'Jane')
<p>Hello, Jane!</p>
@else
<p>Hello, Stranger!</p>
@endif
Loops
Blade cũng hỗ trợ các cấu trúc lặp như for
, foreach
, forelse
, và while
:
<!-- resources/views/home.blade.php -->
@foreach ($users as $user)
<p>This is user {{ $user->name }}</p>
@endforeach
3. Echoing Data
Blade cung cấp cú pháp đơn giản để hiển thị dữ liệu từ các biến PHP:
<!-- resources/views/home.blade.php -->
<p>{{ $name }}</p>
<p>{{ $age }}</p>
Bạn cũng có thể sử dụng hàm @php
để nhúng mã PHP trực tiếp vào file Blade:
<!-- resources/views/home.blade.php -->
@php
$name = 'John';
@endphp
<p>{{ $name }}</p>
4. Escape HTML
Blade sẽ tự động escape các biến để bảo vệ ứng dụng của bạn khỏi các lỗ hổng XSS. Nếu bạn muốn hiển thị dữ liệu mà không escape, bạn có thể sử dụng cú pháp sau:
<!-- resources/views/home.blade.php -->
{!! $unescapedData !!}
5. Kế Thừa Layouts
Blade cung cấp cơ chế kế thừa layout giúp bạn tạo ra các template có cấu trúc lặp lại một cách dễ dàng.
Tạo Layout
<!-- resources/views/layouts/app.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>@yield('title')</title>
</head>
<body>
<div class="container">
@yield('content')
</div>
</body>
</html>
Sử Dụng Layout
<!-- resources/views/home.blade.php -->
@extends('layouts.app')
@section('title', 'Home Page')
@section('content')
<h1>Welcome to My Laravel Application</h1>
@endsection
6. Bao Gồm Các View Con (Including Sub-Views)
Bạn có thể chia nhỏ view thành các phần nhỏ hơn và bao gồm chúng vào view chính:
<!-- resources/views/includes/header.blade.php -->
<header>
<h1>Header Content</h1>
</header>
<!-- resources/views/home.blade.php -->
@include('includes.header')
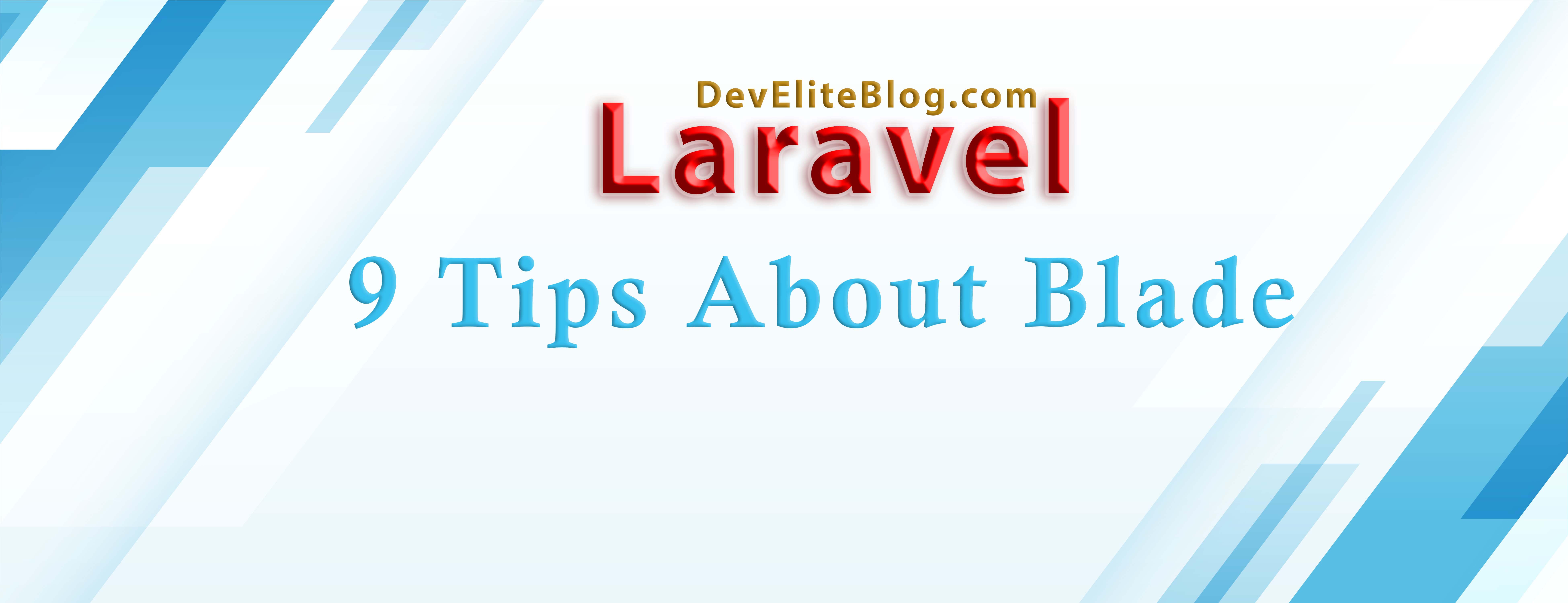
9 Tips about Blade
Author: | ADMIN |
---|
# 9 Tips about Blade
1. Sử dụng forelse
thay vì foreach
Trong Laravel Blade, forelse
là một cấu trúc vòng lặp hữu ích khi bạn muốn xử lý trường hợp một mảng là rỗng. Nó giúp bạn lặp qua một mảng và cung cấp một cấu trúc điều kiện để hiển thị nội dung khi mảng rỗng.
ví dụ:
<!-- resources/views/users.blade.php -->
@foreach ($users as $user)
<p>This is user {{ $user->name }}</p>
@endforeach
@if ($users->isEmpty())
<p>No users found.</p>
@endif
// Replace
<!-- resources/views/users.blade.php -->
@forelse ($users as $user)
<p>This is user {{ $user->name }}</p>
@empty
<p>No users found.</p>
@endforelse
Giải Thích
@forelse ($users as $user)
: Nếu mảng$users
không rỗng, vòng lặp này sẽ lặp qua từng phần tử trong mảng.@empty
: Nếu mảng$users
rỗng, đoạn mã trong khối@empty
sẽ được thực thi.@endforelse
: Kết thúc vòng lặpforelse
.
Laravel cung cấp các directive @auth
và @guest
trong Blade để giúp bạn kiểm tra trạng thái xác thực của người dùng một cách dễ dàng. Đây là hai directive rất hữu ích khi bạn cần hiển thị nội dung khác nhau dựa trên việc người dùng đã đăng nhập hay chưa.
2.1. Directive @auth
Directive @auth
được sử dụng để kiểm tra xem người dùng hiện tại có được xác thực hay không. Nếu người dùng đã đăng nhập, nội dung bên trong directive này sẽ được hiển thị.
Cú Pháp
@auth
<!-- Nội dung dành cho người dùng đã đăng nhập -->
@endauth
Ví Dụ
<!-- resources/views/welcome.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Welcome Page</title>
</head>
<body>
<h1>Welcome to My Laravel Application</h1>
@auth
<p>Welcome back, {{ Auth::user()->name }}!</p>
<a href="{{ route('logout') }}">Logout</a>
@endauth
</body>
</html>
2.2. Directive @guest
Directive @guest
được sử dụng để kiểm tra xem người dùng hiện tại có chưa được xác thực hay không. Nếu người dùng chưa đăng nhập, nội dung bên trong directive này sẽ được hiển thị.
Cú Pháp
@guest
<!-- Nội dung dành cho người dùng chưa đăng nhập -->
@endguest
Ví Dụ
<!-- resources/views/welcome.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Welcome Page</title>
</head>
<body>
<h1>Welcome to My Laravel Application</h1>
@guest
<p>Please <a href="{{ route('login') }}">login</a> to access more features.</p>
<a href="{{ route('register') }}">Register</a>
@endguest
</body>
</html>
Trong các file Blade, bạn có thể sử dụng auth()->user()
để hiển thị thông tin người dùng hiện tại.
<!-- resources/views/profile.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Profile Page</title>
</head>
<body>
<h1>Profile</h1>
@if(auth()->check())
<p>Name: {{ auth()->user()->name }}</p>
<p>Email: {{ auth()->user()->email }}</p>
@else
<p>Please <a href="{{ route('login') }}">login</a> to view your profile.</p>
@endif
</body>
</html>
Các Phương Thức Hữu Ích Khác
auth()->check()
: Kiểm tra xem có người dùng nào đã đăng nhập hay không.auth()->id()
: Lấy ID của người dùng đã đăng nhập.
Carbon cung cấp nhiều phương thức để định dạng ngày và giờ, bao gồm toFormattedDateString
, diffForHumans
, và nhiều hơn nữa.
<p>Created at: {{ $post->created_at->toFormattedDateString() }}</p>
<p>Created at: {{ $post->created_at->diffForHumans() }}</p>
<p>Created at: {{ $post->created_at->format('l, d F Y H:i') }}</p>
Bạn có thể tạo một custom Blade directive để định dạng ngày và giờ một cách nhất quán.
Tạo Custom Blade Directive
Trong AppServiceProvider
, bạn có thể đăng ký một custom Blade directive.
<?php
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use Illuminate\Support\Facades\Blade;
use Carbon\Carbon;
class AppServiceProvider extends ServiceProvider
{
public function boot()
{
Blade::directive('datetime', function ($expression) {
return "<?php echo ($expression)->format('d/m/Y H:i'); ?>";
});
}
public function register()
{
//
}
}
Sử Dụng Custom Blade Directive
<!-- resources/views/show.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Show Date</title>
</head>
<body>
<h1>Show Date</h1>
<p>Created at: @datetime($post->created_at)</p>
</body>
</html>
Trong Laravel, phương thức Route::view
cung cấp một cách tiện lợi để định nghĩa các tuyến đường (route) mà không cần một controller. Nó rất hữu ích cho các trang tĩnh hoặc các trang có ít logic.
Cú Pháp Route::view
Cú pháp cơ bản của Route::view
như sau:
Route::view($uri, $view, $data = []);
$uri
: URL của tuyến đường.$view
: Tên của view sẽ được trả về.$data
: (Tùy chọn) Mảng dữ liệu sẽ được truyền tới view.
Ví Dụ
Dưới đây là một số ví dụ về cách sử dụng Route::view
trong tệp routes/web.php
.
1. Định Nghĩa Một Tuyến Đường Đơn Giản
Giả sử bạn có một view tĩnh tên là welcome.blade.php
trong thư mục resources/views
.
Route::view('/welcome', 'welcome');
Khi người dùng truy cập vào URL /welcome
, họ sẽ thấy nội dung của view welcome.blade.php
.
2. Truyền Dữ Liệu Tới View
Bạn cũng có thể truyền dữ liệu tới view bằng cách sử dụng tham số thứ ba của Route::view
.
Route::view('/about', 'about', ['name' => 'Laravel']);
Trong view about.blade.php
, bạn có thể truy cập dữ liệu này như sau:
<!-- resources/views/about.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>About</title>
</head>
<body>
<h1>About</h1>
<p>This application is built with {{ $name }}.</p>
</body>
</html>
Laravel cung cấp các trang lỗi mặc định cho các mã lỗi HTTP khác nhau, chẳng hạn như 404 (Not Found), 500 (Internal Server Error), v.v. Tuy nhiên, bạn có thể tùy chỉnh các trang lỗi này để phù hợp với giao diện và trải nghiệm người dùng của ứng dụng của mình.
Để tùy chỉnh các trang lỗi, Laravel cung cấp một cách dễ dàng để xuất bản các file view lỗi mặc định vào thư mục views của bạn bằng lệnh php artisan vendor:publish
.
Bước 1: Xuất Bản Các Trang Lỗi
Bạn có thể sử dụng lệnh php artisan vendor:publish --tag=laravel-errors
để xuất bản các trang lỗi mặc định vào thư mục resources/views/errors
của dự án của bạn.
Lệnh
php artisan vendor:publish --tag=laravel-errors
Lệnh này sẽ tạo các file view lỗi trong thư mục resources/views/errors
của dự án của bạn.
Bước 2: Tùy Chỉnh Các Trang Lỗi
Sau khi các file view lỗi đã được xuất bản, bạn có thể tùy chỉnh chúng theo ý muốn của mình. Mỗi file đại diện cho một mã lỗi HTTP cụ thể. Ví dụ, bạn sẽ có các file như 404.blade.php
, 500.blade.php
, v.v.
Ví Dụ Tùy Chỉnh Trang 404
<!-- resources/views/errors/404.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Page Not Found</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
padding: 50px;
}
h1 {
font-size: 50px;
}
p {
font-size: 20px;
}
a {
color: #3490dc;
text-decoration: none;
}
</style>
</head>
<body>
<h1>404</h1>
<p>Sorry, the page you are looking for could not be found.</p>
<p><a href="{{ url('/') }}">Go to Homepage</a></p>
</body>
</html>
Bước 3: Kiểm Tra Các Trang Lỗi
Sau khi tùy chỉnh các trang lỗi, bạn có thể kiểm tra chúng bằng cách truy cập vào các URL không tồn tại hoặc gây ra các lỗi khác nhau trong ứng dụng của bạn. Ví dụ, truy cập một URL không tồn tại để kiểm tra trang 404.
Tóm Tắt
- Xuất bản các trang lỗi: Sử dụng lệnh
php artisan vendor:publish --tag=laravel-errors
để xuất bản các file view lỗi mặc định.- Tùy chỉnh các trang lỗi: Chỉnh sửa các file trong
resources/views/errors
để phù hợp với giao diện của ứng dụng của bạn.- Kiểm tra các trang lỗi: Truy cập các URL không tồn tại hoặc gây ra các lỗi khác để xem các trang lỗi tùy chỉnh của bạn.
Việc tùy chỉnh các trang lỗi giúp bạn tạo ra một trải nghiệm người dùng nhất quán và chuyên nghiệp hơn, ngay cả khi có lỗi xảy ra trong ứng dụng của bạn.
Trong Laravel, lệnh Artisan view:clear
được sử dụng để xóa bộ nhớ cache của các file view đã được biên dịch. Đây là một lệnh hữu ích khi bạn thực hiện các thay đổi trong view và muốn chắc chắn rằng ứng dụng của bạn sẽ sử dụng các file view mới nhất mà không bị ảnh hưởng bởi các phiên bản đã được cache.
Cách Sử Dụng view:clear
Lệnh
Bạn có thể chạy lệnh này từ terminal bằng cách sử dụng:
php artisan view:clear
Kết Quả
Lệnh này sẽ xóa tất cả các file view đã được biên dịch và lưu trữ trong thư mục storage/framework/views
. Sau khi lệnh được thực thi, Laravel sẽ biên dịch lại các file view khi chúng được yêu cầu lần tiếp theo.
Khi Nào Nên Sử Dụng view:clear
- Khi Phát Triển: Nếu bạn thấy rằng các thay đổi bạn thực hiện trong view không được áp dụng, bạn có thể chạy lệnh này để xóa bộ nhớ cache và đảm bảo rằng các thay đổi sẽ được nhìn thấy.
- Khi Triển Khai: Sau khi triển khai ứng dụng lên môi trường production, bạn có thể chạy lệnh này để xóa bộ nhớ cache cũ và buộc Laravel biên dịch lại các view với các thay đổi mới nhất.
Trong Laravel, helper asset()
được sử dụng để tạo URL tuyệt đối tới các tài nguyên công cộng (public assets) như hình ảnh, CSS, JavaScript, v.v. Helper này rất hữu ích để đảm bảo rằng các đường dẫn tới tài nguyên của bạn luôn chính xác, bất kể ứng dụng của bạn đang chạy trên môi trường nào.
asset($path, $secure = null)
$path
: Đường dẫn tới tài nguyên tính từ thư mụcpublic
.$secure
: Tham số tùy chọn, nếu đặt làtrue
, URL sẽ được tạo với HTTPS. Nếu không, HTTP sẽ được sử dụng.
Meta title là một phần quan trọng trong SEO và trải nghiệm người dùng, hiển thị tiêu đề của trang web trên trình duyệt và trong kết quả tìm kiếm. Trong Laravel Blade, bạn có thể dễ dàng thiết lập và tùy chỉnh meta title cho các trang web của mình.
Cách Thiết Lập Meta Title
Để thiết lập meta title trong Laravel Blade, bạn có thể sử dụng thẻ <title>
trong phần <head>
của HTML.
Sử Dụng @section và @yield
Nếu bạn sử dụng layout trong Laravel Blade, bạn có thể thiết lập meta title bằng cách sử dụng @section
và @yield
.
Layout (layouts/app.blade.php)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>@yield('title')</title>
</head>
<body>
<header>
<h1>My Website</h1>
</header>
<div class="content">
@yield('content')
</div>
</body>
</html>
View (home.blade.php)
@extends('layouts.app')
@section('title', 'Home Page')
@section('content')
<h2>Welcome to the Home Page</h2>
<p>This is the home page of the website.</p>
@endsection
Sử Dụng @push và @stack
Một cách khác để thiết lập meta title là sử dụng @push
và @stack
.
Layout (layouts/app.blade.php)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>@stack('title')</title>
</head>
<body>
<header>
<h1>My Website</h1>
</header>
<div class="content">
@yield('content')
</div>
</body>
</html>
View (home.blade.php)
@extends('layouts.app')
@push('title')
Home Page
@endpush
@section('content')
<h2>Welcome to the Home Page</h2>
<p>This is the home page of the website.</p>
@endsection
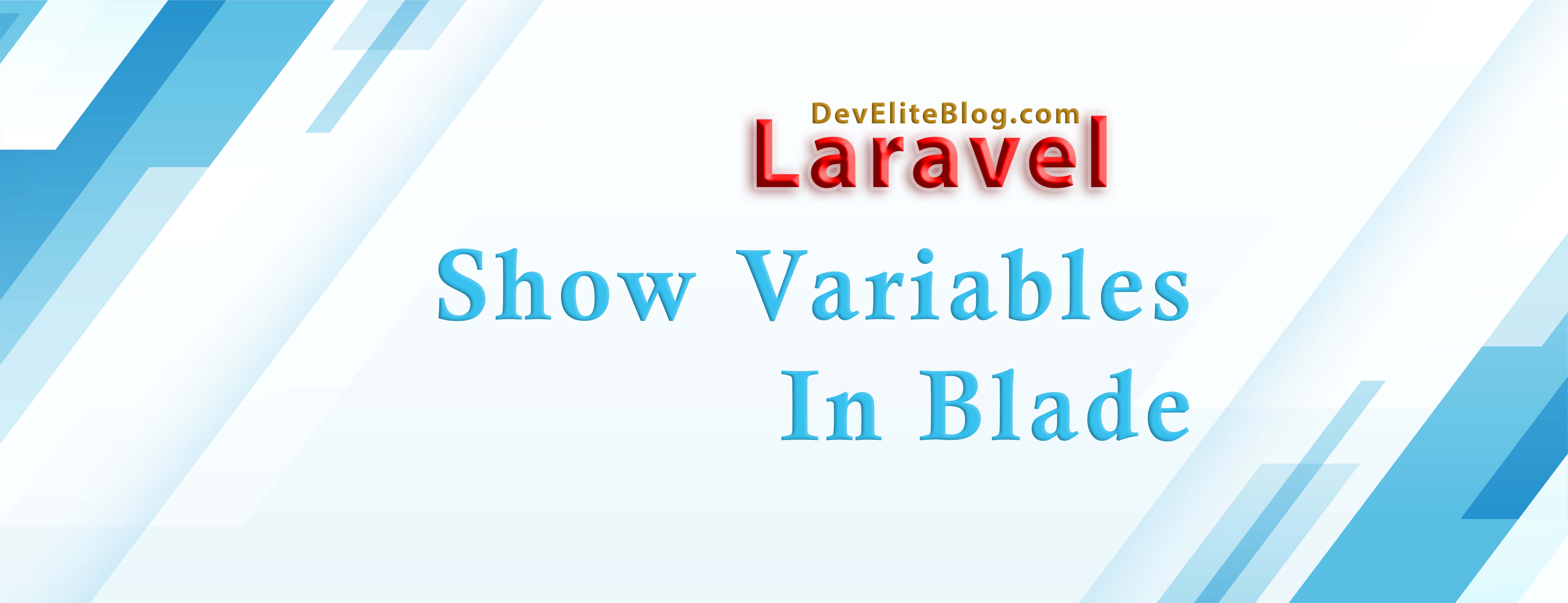
Hiển thị giá trị trong Blade
Author: | ADMIN |
---|
# Hiển Thị Biến Trong Blade
Trong Laravel Blade, việc hiển thị biến rất đơn giản và trực quan. Blade cung cấp cú pháp dễ đọc và sử dụng để nhúng các biến PHP vào trong HTML.
Cú Pháp Cơ Bản
Sử Dụng Cặp Dấu Ngoặc Nhanh {{ }}
Để hiển thị giá trị của một biến trong Blade, bạn chỉ cần sử dụng cặp dấu ngoặc nhanh {{ }}
.
Ví Dụ
Giả sử bạn có một biến $name
được truyền từ controller đến view:
public function show()
{
$name = 'John Doe';
return view('greeting', compact('name'));
}
Trong file view greeting.blade.php
, bạn có thể hiển thị giá trị của biến $name
như sau:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Greeting Page</title>
</head>
<body>
<h1>Hello, {{ $name }}!</h1>
</body>
</html>
Escape HTML Đầu Vào
Khi sử dụng cặp dấu ngoặc nhanh {{ }}
, Blade sẽ tự động escape (chuyển đổi các ký tự đặc biệt thành các thực thể HTML) để bảo vệ chống lại các cuộc tấn công XSS.
Ví Dụ
<?php $name = '<script>alert("XSS")</script>'; ?>
<h1>Hello, {{ $name }}!</h1>
Kết quả hiển thị sẽ là:
<h1>Hello, <script>alert("XSS")</script>!</h1>
Hiển Thị Biến Mà Không Escape HTML
Nếu bạn muốn hiển thị giá trị của biến mà không escape HTML, bạn có thể sử dụng cú pháp {!! !!}
.
<?php $name = '<strong>John Doe</strong>'; ?>
<h1>Hello, {!! $name !!}!</h1>
Kết quả hiển thị sẽ là:
<h1>Hello, <strong>John Doe</strong>!</h1>
Hiển Thị Các Giá Trị Mặc Định
Bạn có thể hiển thị các giá trị mặc định khi biến không tồn tại hoặc rỗng bằng cách sử dụng toán tử null coalescing ??
.
Ví Dụ
<h1>Hello, {{ $name ?? 'Guest' }}!</h1>
Nếu biến $name
không tồn tại hoặc có giá trị là null, chuỗi 'Guest'
sẽ được hiển thị.
Hiển Thị Mảng và Đối Tượng
Bạn có thể truy cập và hiển thị các phần tử của mảng hoặc thuộc tính của đối tượng một cách dễ dàng.
Ví Dụ Mảng
<?php $user = ['name' => 'John Doe', 'email' => 'john@example.com']; ?>
<h1>Name: {{ $user['name'] }}</h1>
<p>Email: {{ $user['email'] }}</p>
Ví Dụ Đối Tượng
<?php $user = (object) ['name' => 'John Doe', 'email' => 'john@example.com']; ?>
<h1>Name: {{ $user->name }}</h1>
<p>Email: {{ $user->email }}</p>
Tóm Tắt
- Cú pháp cơ bản: Sử dụng
{{ $variable }}
để hiển thị giá trị của biến và tự động escape HTML.- Không escape HTML: Sử dụng
{!! $variable !!}
để hiển thị giá trị của biến mà không escape HTML.- Giá trị mặc định: Sử dụng
{{ $variable ?? 'default' }}
để hiển thị giá trị mặc định khi biến không tồn tại hoặc rỗng.- Mảng và đối tượng: Truy cập và hiển thị các phần tử của mảng hoặc thuộc tính của đối tượng bằng cú pháp
{{ $array['key'] }}
và{{ $object->property }}
.Việc sử dụng các cú pháp này giúp bạn dễ dàng và an toàn khi hiển thị dữ liệu trong các view Blade của Laravel.