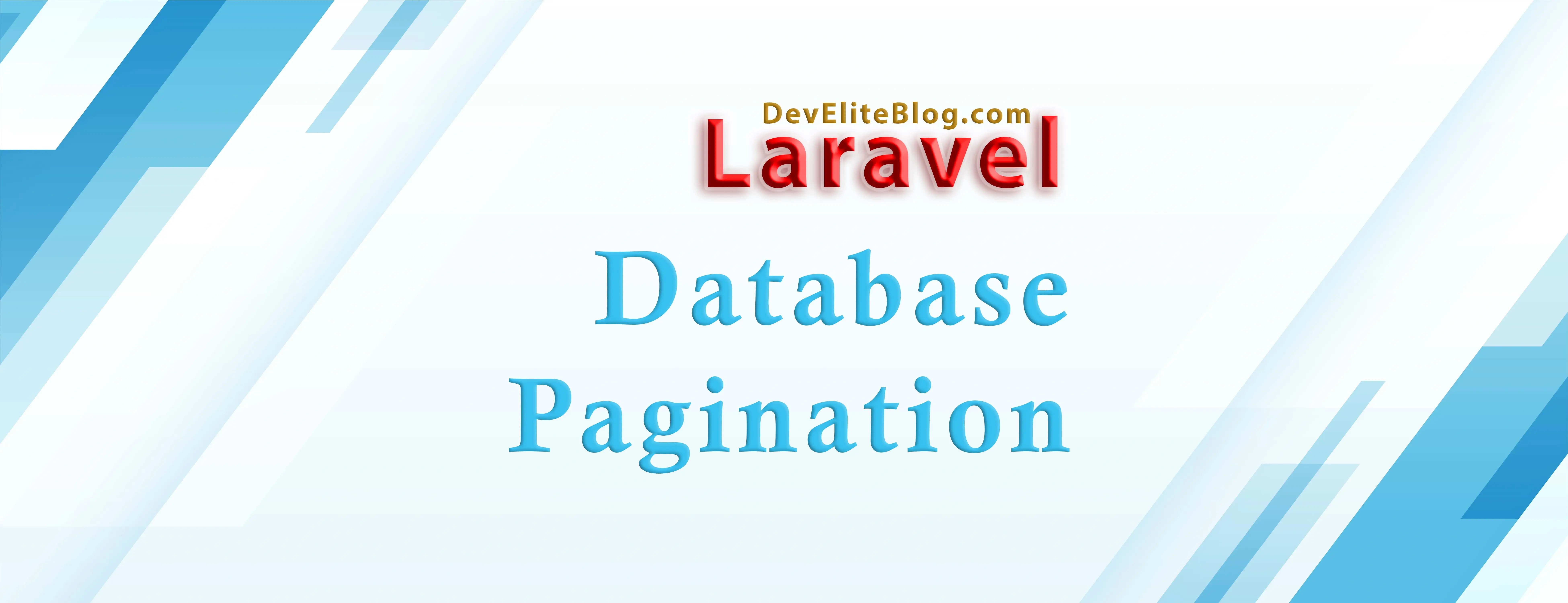
Database: Pagination
01.08.2024
Author: | ADMIN |
---|
Laravel cung cấp nhiều cách để phân trang dữ liệu dễ dàng. Bài viết này hướng dẫn cách sử dụng paginate(), tùy chỉnh URL, và tận dụng các phương thức Paginator để tối ưu hóa trải nghiệm người dùng.
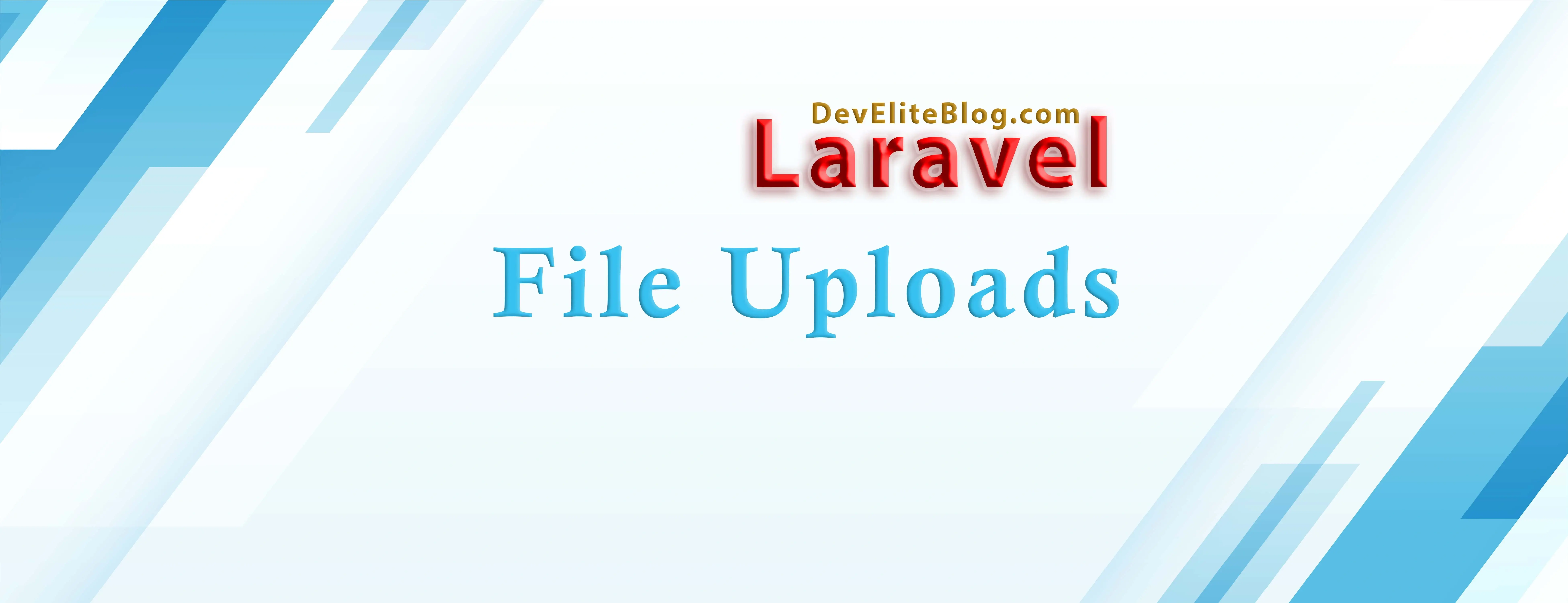
File Uploads
01.08.2024
Author: | ADMIN |
---|
Tìm hiểu cách xử lý file uploads trong Laravel: từ lưu trữ, đặt tên, thiết lập quyền truy cập, đến xóa file và tạo form tải lên. Hướng dẫn chi tiết kèm code mẫu giúp bạn triển khai dễ dàng.
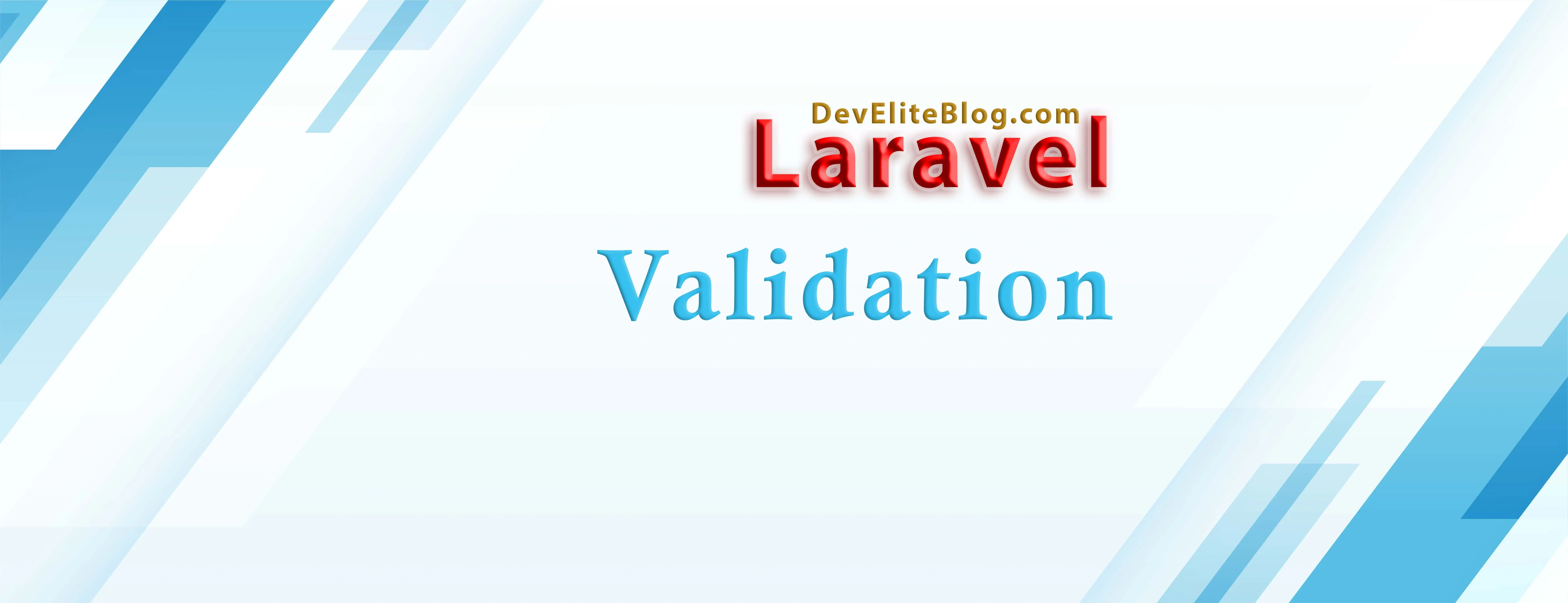
Validation
01.08.2024
Author: | ADMIN |
---|
Tìm hiểu cách sử dụng Laravel Validation để kiểm tra dữ liệu, tối ưu form và xử lý lỗi hiệu quả. Hướng dẫn từ cơ bản đến nâng cao, giúp bạn viết code Laravel chuyên nghiệp hơn! 🚀
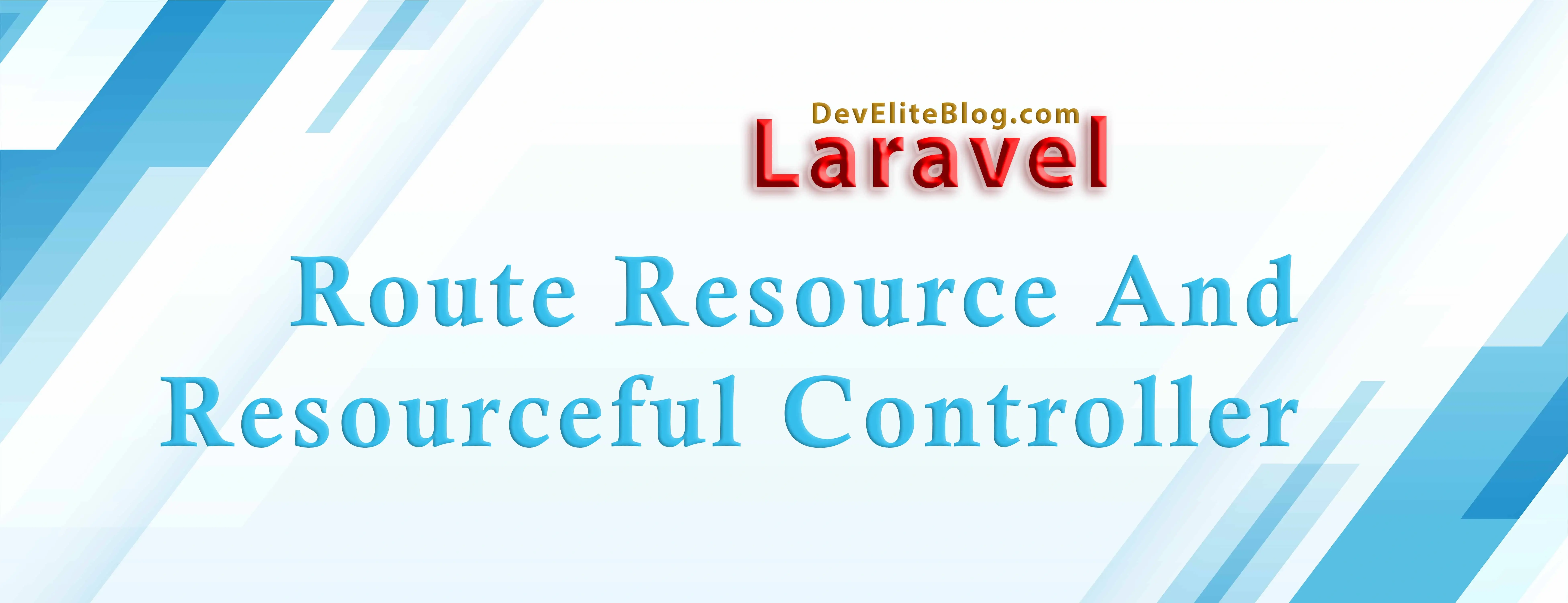
Route Resource and Resourceful Controllers
01.08.2024
Author: | ADMIN |
---|
Tìm hiểu cách sử dụng Resource Controllers trong Laravel để tối ưu hóa CRUD, xử lý Soft Deletes, Nested Resources và tùy chỉnh phản hồi khi model không tồn tại.
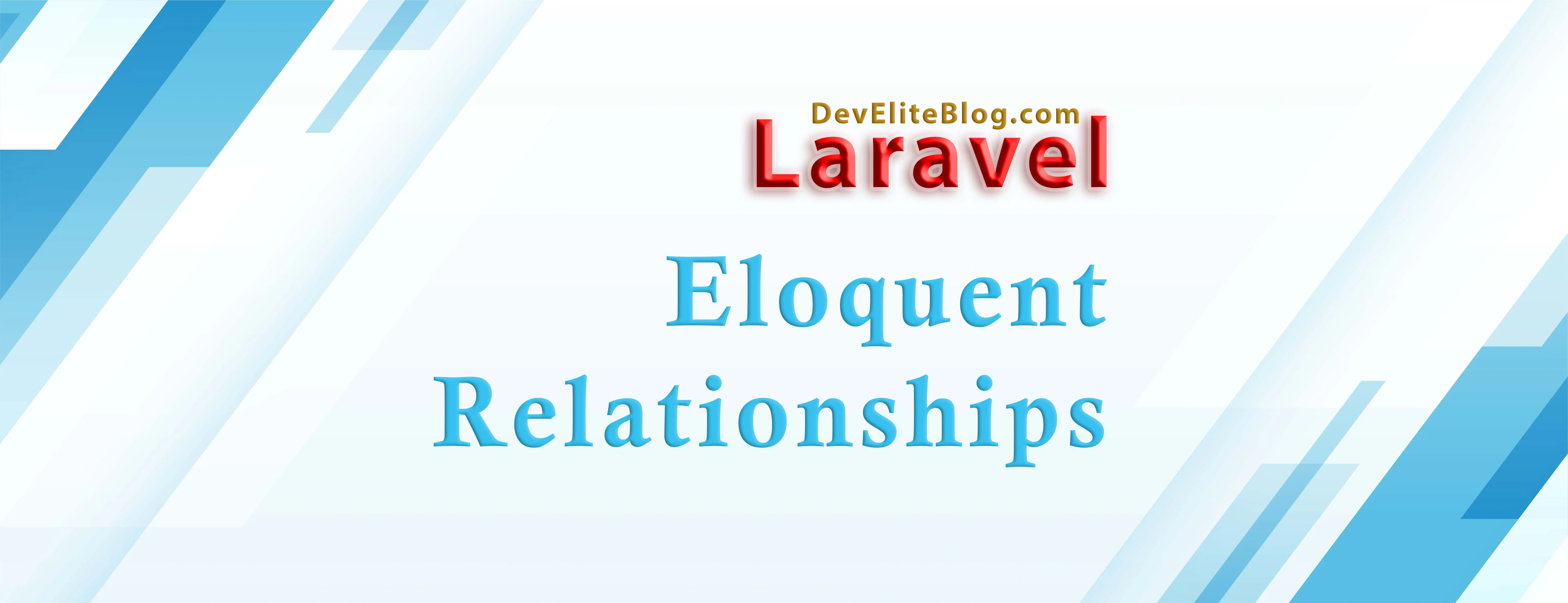
Các Mối Quan Hệ Trong Eloquent
01.08.2024
Author: | ADMIN |
---|
Tìm hiểu cách thiết lập và sử dụng các mối quan hệ trong Eloquent Laravel, giúp quản lý dữ liệu dễ dàng và linh hoạt hơn.
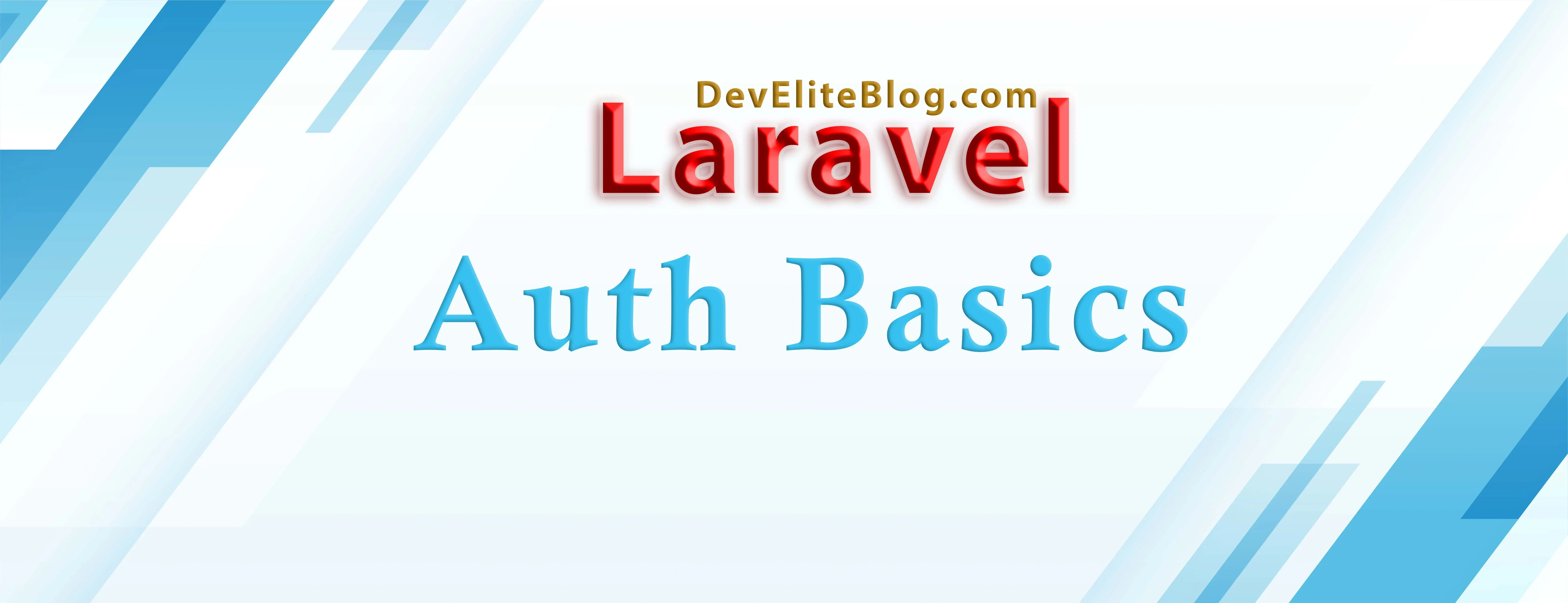
Auth Basics
01.08.2024
Author: | ADMIN |
---|
Laravel cung cấp hệ thống xác thực mạnh mẽ với UI scaffolding, middleware bảo vệ route, xác nhận mật khẩu bổ sung và quy tắc mật khẩu Fortify. Hướng dẫn chi tiết giúp bạn triển khai bảo mật nhanh chóng!
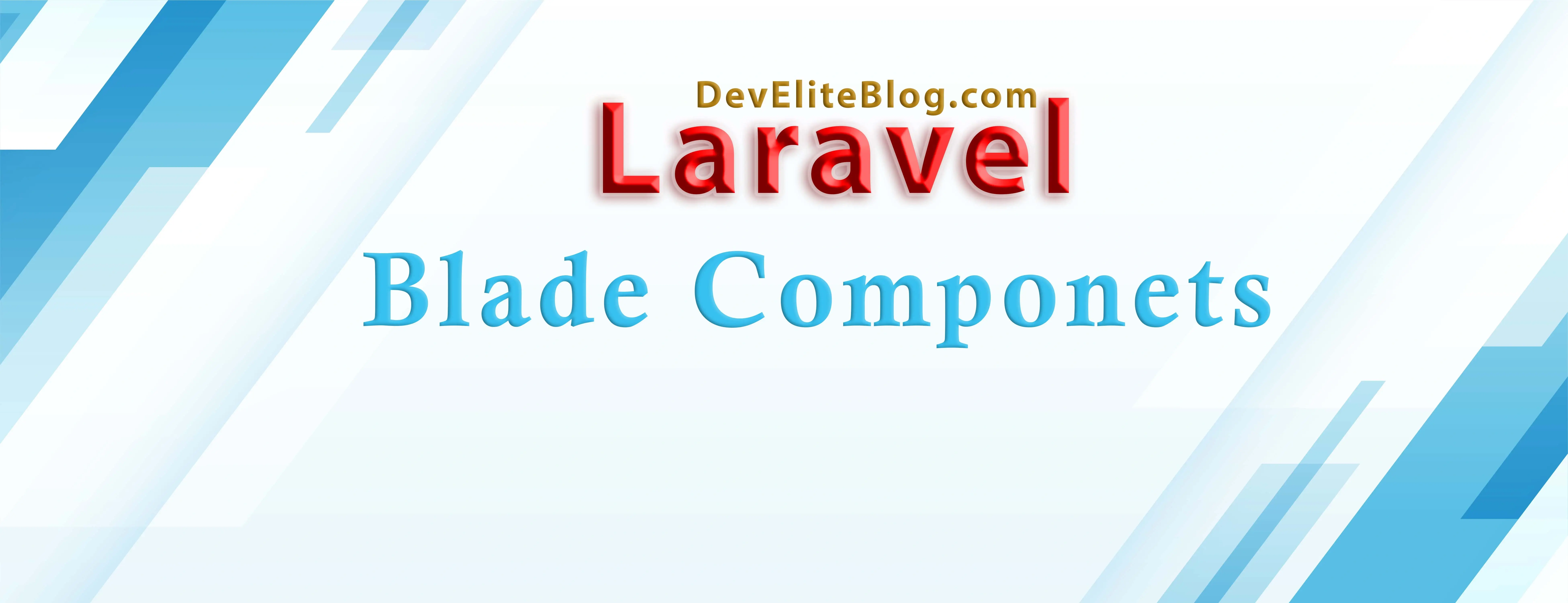
Blade Components
01.08.2024
Author: | ADMIN |
---|
Laravel Blade Components giúp bạn tạo giao diện tái sử dụng, truyền dữ liệu linh hoạt và sử dụng slots thông minh. Hướng dẫn chi tiết giúp mã nguồn sạch hơn, dễ bảo trì và phát triển nhanh chóng!
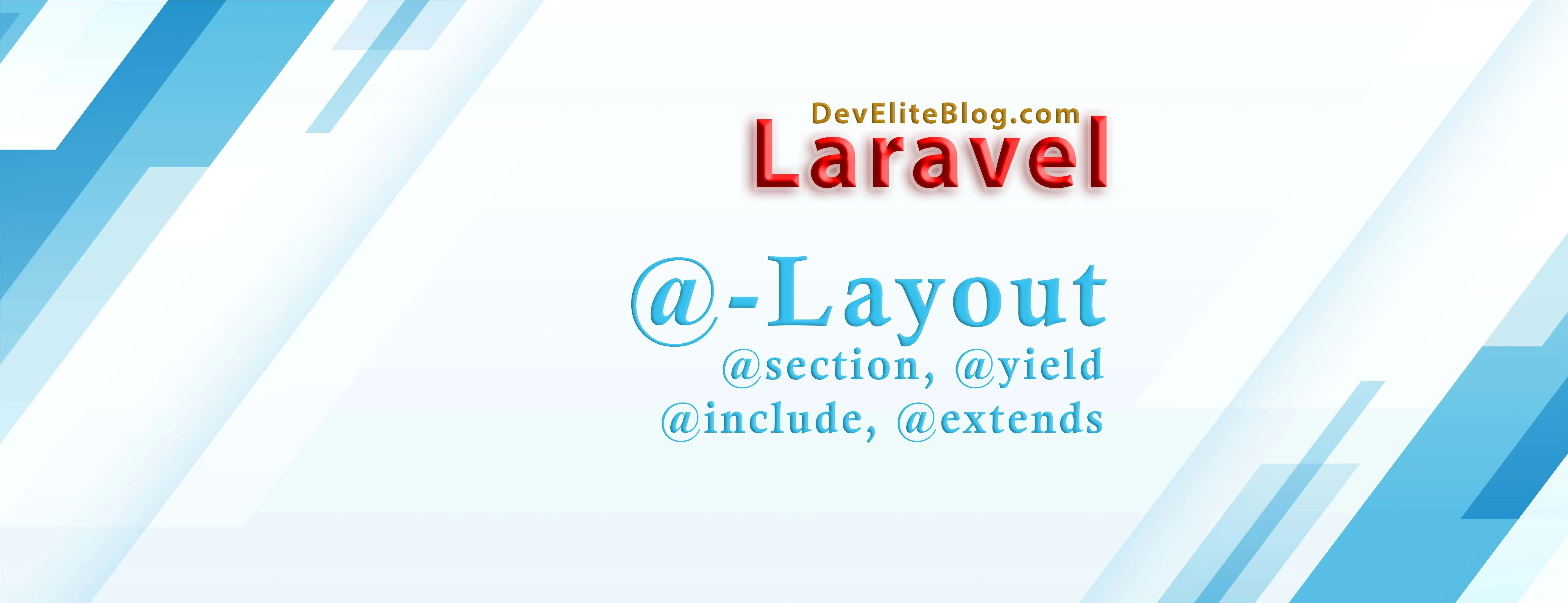
Layout: @include, @extends, @section, @yield
01.08.2024
Author: | ADMIN |
---|
Hướng dẫn chi tiết về @include, @extends, @section và @yield trong Laravel Blade. Tối ưu hóa tái sử dụng giao diện, tổ chức mã rõ ràng, giúp phát triển và bảo trì ứng dụng dễ dàng hơn!
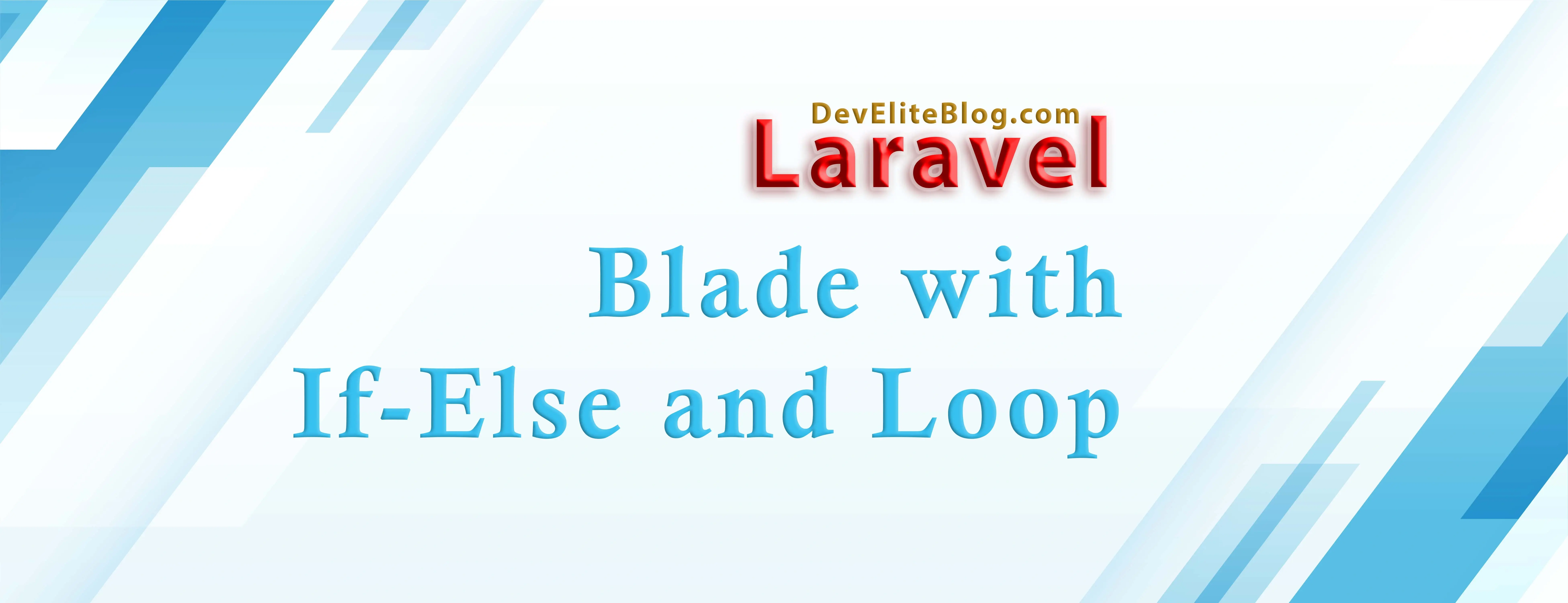
Cấu Trúc Điều Kiện và Vòng Lặp Trong Blade
01.08.2024
Author: | ADMIN |
---|
Khám phá các cấu trúc điều kiện và vòng lặp trong Laravel Blade. Tận dụng @if, @foreach, @forelse để hiển thị dữ liệu linh hoạt, giúp mã nguồn dễ đọc, sạch sẽ và tối ưu hơn!